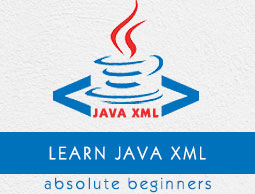
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
AttachmentPart getRawContent() Method
Description
The Javax.xml.soap.AttachmentPart.getRawContent() method gets the content of this AttachmentPart object as an InputStream as if a call had been made to getContent and no DataContentHandler had been registered for the content-type of this AttachmentPart.
Declaration
Following is the declaration for javax.xml.soap.AttachmentPart.getRawContent() method
public abstract InputStream getRawContent()
Parameters
NA
Return Value
This method returns an InputStream from which the raw data contained by the AttachmentPart can be accessed.
Exception
SOAPException − if there is no content set into this AttachmentPart object or if there was a data transformation error.
Example
The following example shows the usage of javax.xml.soap.AttachmentPart.getRawContent() method.
package com.tutorialspoint; import com.sun.xml.internal.messaging.saaj.util.Base64; import com.sun.xml.internal.messaging.saaj.util.ByteInputStream; import java.io.InputStream; import java.util.Iterator; import javax.activation.DataHandler; import javax.xml.soap.*; public class AttachmentPartDemo { public static void main(String[] args) { try { // create a new SOAPMessage SOAPMessage message = MessageFactory.newInstance().createMessage(); // create a string as a new attachment String attachment = "This is an attachment"; // get the data handler and assign the attachment DataHandler handler = new DataHandler(attachment, "HandlerHeader"); // create the attachment part AttachmentPart attachPart = message.createAttachmentPart(); // add raw content byte[] encoded = Base64.encode(attachment.getBytes()); ByteInputStream bis = new ByteInputStream(encoded, 0, encoded.length); attachPart.setRawContent(bis, "plain/text"); // add the attachment part in the message message.addAttachmentPart(attachPart); // read the raw content InputStream content = attachPart.getRawContent(); for (int i = 0; i < content.available(); i++) { System.out.print("" + (char) content.read()); } } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
VGhpcyBpcyBhbi
javax_xml_soap_attachmentpart.htm
Advertisements
To Continue Learning Please Login