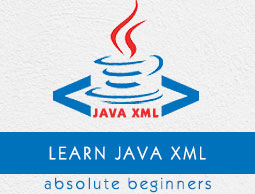
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
AttachmentPart getBase64Content() Method
Description
The Javax.xml.soap.AttachmentPart.getBase64Content() method returns an InputStream which can be used to obtain the content of AttachmentPart as Base64 encoded character data, this method would base64 encode the raw bytes of the attachment and return.
Declaration
Following is the declaration for javax.xml.soap.AttachmentPart.getBase64Content() method
public abstract InputStream getBase64Content()
Parameters
NA
Return Value
This method returns an InputStream from which the Base64 encoded AttachmentPart can be read.
Exception
SOAPException − if there is no content set into this AttachmentPart object or if there was a data transformation error.
Example
The following example shows the usage of javax.xml.soap.AttachmentPart.getBase64Content() method.
package com.tutorialspoint; import com.sun.xml.internal.messaging.saaj.util.Base64; import com.sun.xml.internal.messaging.saaj.util.ByteInputStream; import java.io.InputStream; import javax.xml.soap.*; public class AttachmentPartDemo { public static void main(String[] args) { try { // create a new SOAPMessage SOAPMessage message = MessageFactory.newInstance().createMessage(); // create a string as a new attachment String attachment = "This is an attachment"; // create the attachment part AttachmentPart attachPart = message.createAttachmentPart(); // encode attachment and add it as AttachmentPart byte[] encoded = Base64.encode(attachment.getBytes()); ByteInputStream bis = new ByteInputStream(encoded, 0, encoded.length); attachPart.setBase64Content(bis, "plain/text"); // add the attachment part in the message message.addAttachmentPart(attachPart); // get the inputstream for attachPart InputStream is = attachPart.getBase64Content(); // print the content for (int i = 0; i < is.available(); i++) { System.out.print("" + (char) is.read()); } } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
VGhpcyBpcyBhbi
javax_xml_soap_attachmentpart.htm
Advertisements
To Continue Learning Please Login