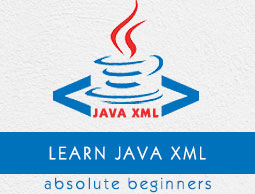
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
ValidationEventCollector.hasEvents() Method
Description
The Javax.xml.bind.util.ValidationEventCollector.hasEvents() method returns true if this event collector contains at least one ValidationEvent.
Declaration
Following is the declaration for javax.xml.bind.util.ValidationEventCollector.hasEvents() method
public boolean hasEvents()
Parameters
NA
Return Value
This method returns true if this event collector contains at least one ValidationEvent, false otherwise
Exception
NA
Example
The following example shows the usage of javax.xml.bind.util.ValidationEventCollector.hasEvents() method. To proceed, consider the following Student class which will be used to have objects for marshalling purpose −
package com.tutorialspoint; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Student{ String name; int age; int id; public String getName(){ return name; } @XmlElement public void setName(String name){ this.name = name; } public int getAge(){ return age; } @XmlElement public void setAge(int age){ this.age = age; } public int getId(){ return id; } @XmlAttribute public void setId(int id){ this.id = id; } }
Now let us create main class which will be used to unmarshal ie. convert Student.xml file into an Student object. This example unmarshals the Student.xml file and prints exception details if any error occurs inside the XML
package com.tutorialspoint; import java.io.File; import javax.xml.XMLConstants; import javax.xml.bind.JAXBContext; import javax.xml.bind.Unmarshaller; import javax.xml.bind.util.ValidationEventCollector; import javax.xml.validation.Schema; import javax.xml.validation.SchemaFactory; public class ValidationEventCollectorDemo { public static void main(String[] args) { Schema mySchema; String SCHEMA_NS_URI = XMLConstants.W3C_XML_SCHEMA_NS_URI; // create SchemaFactory SchemaFactory sf = SchemaFactory.newInstance(SCHEMA_NS_URI); // validation Event collector ValidationEventCollector vec = new ValidationEventCollector(); try{ // parse schema file and return as schema object mySchema=sf.newSchema(new File("Student.xsd")); // create JAXBContext object JAXBContext jc = JAXBContext.newInstance(Student.class); // create umarshaller to unmarshal xml to object Unmarshaller u = jc.createUnmarshaller(); // set schema object u.setSchema(mySchema); // set event handler u.setEventHandler( vec ); // create student object Student s = (Student)u.unmarshal(new File("Student.xml")); }catch(Exception e){ }finally{ if( vec != null && vec.hasEvents() ){ System.out.print("Validation events exists: "+vec.hasEvents()); } } } }
For unmarshalling we must provide XML as input to the main program −
<?xml version = "1.0" encoding = "UTF-8" standalone = "yes"?> <student id = "10"> <age1>12</age1> <name>Malik</name> </student>
Before we proceed for compilation, we need to make sure that that we download JAXB2.xxx.jar and put it in our CLASSPATH. Once setup is ready, let us compile and run the above program, this will produce the following result −
Validation events exists: true
To Continue Learning Please Login