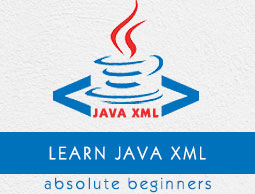
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Java.xml.bind.JAXBElement.isNil() Method
Description
The Java.xml.bind.JAXBElement.isNil() method returns true if this element instance content model is nil.
Declaration
Following is the declaration for java.xml.bind.JAXBElement.isNil() method −
public boolean isNil()
Parameters
NA
Return Value
Returns true if this element instance content model is nil.
Exception
NA
Example
The following example shows the usage of java.xml.bind.JAXBElement.isNil() method. To proceed, consider the following Student class which will be used to have objects for marshalling and unmarshalling purposes −
package com.tutorialspoint; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Student{ String name; int age; int id; public String getName(){ return name; } @XmlElement public void setName(String name){ this.name = name; } public int getAge(){ return age; } @XmlElement public void setAge(int age){ this.age = age; } public int getId(){ return id; } @XmlAttribute public void setId(int id){ this.id = id; } }
Now let us create main class which will show the usage of java.xml.bind.JAXBElement.isNil() method. Here we will create a JAXBElement of a Student object and then get the class of Student. This example checks the content model of JAXBElement object and prints the result.
package com.tutorialspoint; import javax.xml.bind.JAXBElement; import javax.xml.namespace.QName; public class JAXBElementDemo { public static void main(String[] args) { //Create a student object Student student = new Student(); //fill details of the student student.setName("Robert"); student.setId(1); student.setAge(12); //create JAXBElement of type Student //Pass it the student object JAXBElement<Student> jaxbElement = new JAXBElement( new QName(Student.class.getSimpleName()), Student.class, student); //check the content model boolean isContentNil = jaxbElement.isNil(); //print the name System.out.println("Content Nil (jaxElement)?: "+isContentNil); //create JAXBElement of type Student //Pass it a null object JAXBElement<Student> jaxbElement1 = new JAXBElement( new QName(Student.class.getSimpleName()), Student.class, null); //check the content model isContentNil = jaxbElement1.isNil(); //print the result System.out.println("Content Nil (jaxElement1)?: "+isContentNil); } }
Let us compile and run the above program, this will produce the following result −
Content Nil (jaxElement)?: false Content Nil (jaxElement1)?: true
To Continue Learning Please Login