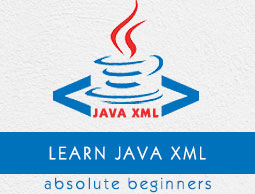
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Javax.xml.bind.JAXBContext.generateSchema() Method
Description
The Javax.xml.bind.JAXBContext.generateSchema(SchemaOutputResolver outputResolver) method generates the schema documents for this context.
Declaration
Following is the declaration for javax.xml.bind.JAXBContext.generateSchema(SchemaOutputResolver outputResolver) method
public void generateSchema(SchemaOutputResolver outputResolver)
Parameters
outputResolver − This object controls the output to which schemas will be sent.
Return Value
The method does not return any value.
Exception
IOException − if SchemaOutputResolver throws an IOException.
UnsupportedOperationException − Calling this method on JAXB 1.0 implementations will throw an UnsupportedOperationException.
Example
The following example shows the usage of javax.xml.bind.JAXBContext.generateSchema(SchemaOutputResolver outputResolver) method. To proceed, consider the following Student class which will be used to have objects for marshalling purpose −
package com.tutorialspoint; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Student{ String name; int age; int id; public String getName(){ return name; } @XmlElement public void setName(String name){ this.name = name; } public int getAge(){ return age; } @XmlElement public void setAge(int age){ this.age = age; } public int getId(){ return id; } @XmlAttribute public void setId(int id){ this.id = id; } }
The program below generates XSD from the Student.java class.
package com.tutorialspoint; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import javax.xml.bind.JAXBContext; import javax.xml.bind.SchemaOutputResolver; public class JAXBContextDemo { public static void main(String[] args) throws IOException { FileInputStream fis = null; try { // create new JAXB context JAXBContext context = JAXBContext.newInstance(Student.class); // create new schema out put resolver SchemaOutputResolver sor = new DemoSchemaOutputResolver(); // generate schema context.generateSchema(sor); // create new file File fi = new File("C://test.txt"); fis = new FileInputStream(fi); int i=0; while((i=fis.read())!=-1) { char c=(char)i; System.out.print(c); } }catch(Exception ex) { ex.printStackTrace(); }finally{ if(fis!=null) fis.close(); } } }
The DemoSchemaOutputResolver class extends to the SchemaOutputResolver. A blank test.txt file is created and referenced to the program where the XSD content will be generated.
package com.tutorialspoint; import java.io.File; import java.io.IOException; import javax.xml.bind.SchemaOutputResolver; import javax.xml.transform.Result; import javax.xml.transform.stream.StreamResult; public class DemoSchemaOutputResolver extends SchemaOutputResolver { @Override public Result createOutput(String namespaceUri, String suggestedFileName) throws IOException { // create new file File file = new File("c://test.txt"); // create stream result StreamResult result = new StreamResult(file); // set system id result.setSystemId(file.toURI().toURL().toString()); // return result return result; } }
Before we proceed for compilation, we need to make sure that that we download JAXB2.xxx.jar and put it in our CLASSPATH. Once setup is ready, let us compile and run the above program, this will produce the following result −
<?xml version = "1.0" encoding = "UTF-8" standalone = "yes"?> <xs:schema version = "1.0" xmlns:xs = "http://www.w3.org/2001/XMLSchema"> <xs:element name = "student" type = "student"/> <xs:complexType name = "student"> <xs:sequence> <xs:element name = "age" type = "xs:int"/> <xs:element name = "name" type="xs:string" minOccurs = "0"/> </xs:sequence> <xs:attribute name = "id" type = "xs:int" use = "required"/> </xs:complexType> </xs:schema>
To Continue Learning Please Login