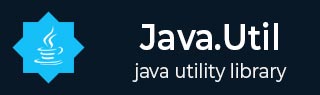
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
java.util.TreeSet.comparator() Method
Description
The comparator() method returns the comparator used to order the elements in this set, or null if this set uses the natural ordering of its elements.
Declaration
Following is the declaration for java.util.TreeSet.comparator() method.
public Comparator<? super E> comparator()
Parameters
NA
Return Value
The method call returns the comparator used to order the elements in this set, or null if this set uses the natural ordering of its elements.
Exception
NA
Example
The following example shows the usage of java.util.TreeSet.comparator() method.
package com.tutorialspoint; import java.util.Iterator; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating TreeSet TreeSet <Integer>tree = new TreeSet<Integer>(); TreeSet <Integer>treecomp = new TreeSet<Integer>(); // adding in the tree tree.add(12); tree.add(13); tree.add(14); tree.add(15); tree.add(16); tree.add(17); // using comparator treecomp = (TreeSet)tree.comparator(); if(treecomp!=null) { for (Integer element : treecomp) System.out.println(element + " "); } else { System.out.println("treecomp value: "+treecomp); System.out.println("So it is using natural ordering"); } } }
Let us compile and run the above program, this will produce the following result.
treecomp value: null So it is using natural ordering
java_util_treeset.htm
Advertisements