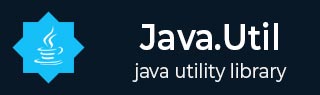
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Properties setProperty(String key,String value) Method
Description
The Java Properties setProperty(String key,String value) method calls the Hashtable method put. Provided for parallelism with the getProperty method. Enforces use of strings for property keys and values. The value returned is the result of the Hashtable call to put.
Declaration
Following is the declaration for java.util.Properties.setProperty() method
public Object setProperty(String key,String value)
Parameters
key − the key to be placed into this property list.
value − the value corresponding to key.
Return Value
This method returns the previous value of the specified key in this property list, or null if it did not have one.
Exception
NA
Updating a Key of Properties Example
The following example shows the usage of java.util.Properties.setProperty() method. We've created a Properties object and add properties using setProperty and put method and then properties object is printed. Using setProperty() method again, we've updated the properties and printed them.
package com.tutorialspoint; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.setProperty("Height", "200"); prop.put("Width", "1500"); // print the list System.out.println("" + prop); // change the properties prop.setProperty("Width", "15"); prop.setProperty("Height", "500"); // print the list System.out.println("" + prop); } }
Output
Let us compile and run the above program, this will produce the following result −
{Height=200, Width=1500} {Height=500, Width=15}
Adding a Key of Properties Example
The following example shows the usage of java.util.Properties.setProperty() method. We've created a Properties object and add properties using setProperty methods and then properties object is printed. Using setProperty() method again, we've updated the properties and printed them.
package com.tutorialspoint; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.setProperty("Height", "200"); prop.setProperty("Width", "1500"); // print the list System.out.println("" + prop); // change the properties prop.setProperty("Width", "15"); prop.setProperty("Height", "500"); // print the list System.out.println("" + prop); } }
Output
Let us compile and run the above program, this will produce the following result −
{Height=200, Width=1500} {Height=500, Width=15}
To Continue Learning Please Login