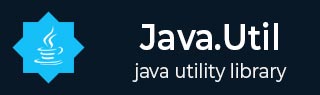
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java - Deque Interface
The Deque interface is provided in java.util package and it extends the Queue interface. It is a linear collection that supports element insertion and removal at both ends. This interface defines methods to access the elements at both ends of the deque. Methods are provided to insert, remove, and examine the element.
Declaration
public interface Deque<E> extends Queue<E>
Deque Methods
Following is the list of the important deque methods that all the implementation classes of the Deque interface implement −
Sr.No. | Method & Description |
---|---|
1 | boolean add(E e)
This method inserts the specified element into the queue represented by this deque (in other words, at the tail of this deque) if it is possible to do so immediately without violating capacity restrictions, returning true upon success and throwing an IllegalStateException if no space is currently available. |
2 | boolean addAll(Collection<? extends E> c)
This method adds all of the elements in the specified collection at the end of this deque, as if by calling addLast(E) on each one, in the order that they are returned by the collection's iterator. |
3 | void addFirst(E e)
This method inserts the specified element at the front of this deque if it is possible to do so immediately without violating capacity restrictions, throwing an IllegalStateException if no space is currently available. |
4 | void addLast(E e)
This method inserts the specified element at the end of this deque if it is possible to do so immediately without violating capacity restrictions, throwing an IllegalStateException if no space is currently available. |
5 | boolean contains(Object o)
This method returns true if this deque contains the specified element. |
6 | Iterator<E> descendingIterator()
This method returns an iterator over the elements in this deque in reverse sequential order. |
7 | E element()
This method retrieves, but does not remove, the head of the queue represented by this deque (in other words, the first element of this deque). |
8 | E getFirst()
This method retrieves, but does not remove, the first element of this deque. |
9 | E getLast()
This method retrieves, but does not remove, the last element of this deque. |
10 | Iterator<E> iterator()
This method returns an iterator over the elements in this deque in proper sequence. |
11 | boolean offer(E e)
This method inserts the specified element into the queue represented by this deque (in other words, at the tail of this deque) if it is possible to do so immediately without violating capacity restrictions, returning true upon success and false if no space is currently available. |
12 | boolean offerFirst(E e)
This method inserts the specified element at the front of this deque unless it would violate capacity restrictions. |
13 | boolean offerLast(E e)
This method inserts the specified element at the end of this deque unless it would violate capacity restrictions. |
14 | E peek()
This method retrieves, but does not remove, the head of the queue represented by this deque (in other words, the first element of this deque), or returns null if this deque is empty. |
15 | E peekFirst()
This method retrieves, but does not remove, the first element of this deque, or returns null if this deque is empty. |
16 | E peekLast()
This method retrieves, but does not remove, the last element of this deque, or returns null if this deque is empty. |
17 | E poll()
This method retrieves and removes the head of the queue represented by this deque (in other words, the first element of this deque), or returns null if this deque is empty. |
18 | E pollFirst()
This method retrieves and removes the first element of this deque, or returns null if this deque is empty. |
19 | E pollLast()
This method retrieves and removes the last element of this deque, or returns null if this deque is empty. |
20 | E pop()
This method pops an element from the stack represented by this deque. |
21 | void push(E e)
This method pushes an element onto the stack represented by this deque (in other words, at the head of this deque) if it is possible to do so immediately without violating capacity restrictions, throwing an IllegalStateException if no space is currently available. |
22 | E remove()
This method retrieves and removes the head of the queue represented by this deque (in other words, the first element of this deque). |
23 | E removeFirst()
This method retrieves and removes the first element of this deque. |
24 | boolean removeFirstOccurrence(Object o)
This method removes the first occurrence of the specified element from this deque. |
25 | E removeLast()
This method retrieves and removes the last element of this deque. |
26 | boolean removeLastOccurrence(Object o)
This method removes the last occurrence of the specified element from this deque. |
27 | int size()
This method returns the number of elements in this deque. |
Methods inherited
This interface inherits methods from the following interfaces −
- java.util.Queue
- java.util.Collection
- java.lang.Iterable
Example
In this example, we're using a Deque instance to show deque add, peek and size operations.
package com.tutorialspoint; import java.util.ArrayDeque; import java.util.Deque; public class DequeDemo { public static void main(String[] args) { Deque<Integer> q = new ArrayDeque<>(); q.add(6); q.add(1); q.add(8); q.add(4); q.add(7); System.out.println("The deque is: " + q); int num1 = q.remove(); System.out.println("The element deleted from the head is: " + num1); System.out.println("The deque after deletion is: " + q); int head = q.peek(); System.out.println("The head of the deque is: " + head); int size = q.size(); System.out.println("The size of the deque is: " + size); } }
Output
The deque is: [6, 1, 8, 4, 7] The element deleted from the head is: 6 The deque after deletion is: [1, 8, 4, 7] The head of the deque is: 1 The size of the deque is: 4
To Continue Learning Please Login