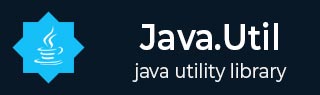
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Hashtable isEmpty() Method
Description
The Java Hashtable isEmpty() method is used to test if this hashtable maps no keys to values.
Declaration
Following is the declaration for java.util.Hashtable.isEmpty() method.
public boolean isEmpty()
Parameters
NA
Return Value
The method call returns 'true' if this hashtable maps no keys to values; false otherwise.
Exception
NA
Checking HashTable of Integer, Integer Pair being Empty or Not Example
The following example shows the usage of Java Hashtable isEmpty() method to check a Hashtable if it is empty or not. We've created a Hashtable object of Integer,Integer pairs. Then few entries are added, table is printed. Then table is checked using isEmpty() method. Using clear() method, table is cleared and printed again and checked again using isEmpty() method.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,Integer> hashtable = new Hashtable<>(); // populate hash table hashtable.put(1, 1); hashtable.put(2, 2); hashtable.put(3, 3); System.out.println("Initial table elements: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); // clear hash table hashtable.clear(); System.out.println("Hashtable elements after clear: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); } }
Output
Let us compile and run the above program, this will produce the following result.
Initial table elements: {3=3, 2=2, 1=1} Is table Empty: false Hashtable elements after clear: {} Is table Empty: true
Checking HashTable of Integer, String Pair being Empty or Not Example
The following example shows the usage of Java Hashtable isEmpty() method to check a Hashtable if it is empty or not. We've created a Hashtable object of Integer,String pairs. Then few entries are added, table is printed. Then table is checked using isEmpty() method. Using clear() method, table is cleared and printed again and checked again using isEmpty() method.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,String> hashtable = new Hashtable<>(); // populate hash table hashtable.put(1, "tutorials"); hashtable.put(2, "point"); hashtable.put(3, "is best"); System.out.println("Initial table elements: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); // clear hash table hashtable.clear(); System.out.println("Hashtable elements after clear: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); } }
Output
Let us compile and run the above program, this will produce the following result.
Initial table elements: {3=is best, 2=point, 1=tutorials} Is table Empty: false Hashtable elements after clear: {} Is table Empty: true
Checking HashTable of Integer, Object Pair being Empty or Not Example
The following example shows the usage of Java Hashtable isEmpty() method to check a Hashtable if it is empty or not. We've created a Hashtable object of Integer,Student pairs. Then few entries are added, table is printed. Then table is checked using isEmpty() method. Using clear() method, table is cleared and printed again and checked again using isEmpty() method.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,Student> hashtable = new Hashtable<>(); // populate hash table hashtable.put(1, new Student(1, "Julie")); hashtable.put(2, new Student(2, "Robert")); hashtable.put(3, new Student(3, "Adam")); System.out.println("Initial table elements: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); // clear hash table hashtable.clear(); System.out.println("Hashtable elements after clear: " + hashtable); System.out.println("Is table Empty: " + hashtable.isEmpty()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Initial table elements: {3=[ 3, Adam ], 2=[ 2, Robert ], 1=[ 1, Julie ]} Is table Empty: false Hashtable elements after clear: {} Is table Empty: true