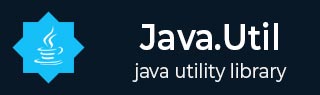
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Hashtable equals() Method
Description
The Java Hashtable equals(Object o) method is used to compare the specified Object with this Hashtable for equality.
Declaration
Following is the declaration for java.util.Hashtable.equals() method.
public boolean equals(Object o)
Parameters
o − This is the object to be compared for equality with this Hashtable.
Return Value
The method call returns 'true' if the specified Object is equal to this Hashtable.
Exception
NA
Checking Equality of HashTables of Integer, Integer Pair Example
The following example shows the usage of Java Hashtable equals() method to check if two Hashtable objects are equal or not. We've created two Hashtable objects of Integer,Integer pairs. Then few entries are added to hashtables and then using equals() method, tables are compared.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,Integer> hashtable = new Hashtable<>(); Hashtable<Integer,Integer> hashtable1 = new Hashtable<>(); // populate hash table hashtable.put(1, 1); hashtable.put(2, 2); hashtable.put(3, 3); // populate hash table hashtable1.put(1, 1); hashtable1.put(2, 2); hashtable1.put(3, 3); System.out.println("hashtables are same: " + hashtable.equals(hashtable1)); } }
Output
Let us compile and run the above program, this will produce the following result.
hashtables are same: true
Checking Equality of HashTables of Integer, String Pair Example
The following example shows the usage of Java Hashtable equals() method to check if two Hashtable objects are equal or not. We've created two Hashtable objects of Integer,String pairs. Then few entries are added to hashtables and then using equals() method, tables are compared.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,String> hashtable = new Hashtable<>(); Hashtable<Integer,String> hashtable1 = new Hashtable<>(); // populate hash table hashtable.put(1, "tutorials"); hashtable.put(2, "point"); hashtable.put(3, "is best"); hashtable1.put(1, "tutorials"); hashtable1.put(2, "point"); hashtable1.put(3, "is best"); System.out.println("hashtables are same: " + hashtable.equals(hashtable1)); } }
Output
Let us compile and run the above program, this will produce the following result.
hashtables are same: true
Checking Equality of HashTables of Integer, Object Pair Example
The following example shows the usage of Java Hashtable equals() method to check if two Hashtable objects are equal or not. We've created two Hashtable objects of Integer,Student pairs. Then few entries are added to hashtables and then using equals() method, tables are compared.
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,Student> hashtable = new Hashtable<>(); Hashtable<Integer,Student> hashtable1 = new Hashtable<>(); // populate hash table hashtable.put(1, new Student(1, "Julie")); hashtable.put(2, new Student(2, "Robert")); hashtable.put(3, new Student(3, "Adam")); // populate hash table hashtable1.put(1, new Student(1, "Julie")); hashtable1.put(2, new Student(2, "Robert")); hashtable1.put(3, new Student(3, "Adam")); System.out.println("hashtables are same: " + hashtable.equals(hashtable1)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int hashCode() { return rollNo + name.hashCode(); } }
Output
Let us compile and run the above program, this will produce the following result.
hashtables are same: true