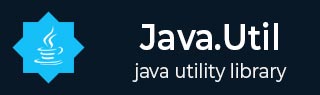
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet spliterator() Method
Description
The Java HashSet spliterator() method is used to get late-binding and fail-fast Spliterator over the elements in this set. The elements are returned in no particular order.
Declaration
Following is the declaration for java.util.HashSet.spliterator() method.
public Spliterator<E> spliterator()
Parameters
NA
Return Value
The method call returns an Spliterator over the elements in this set.
Exception
NA
Getting Spliterator to Iterate Entries of the HashSet of Integers Example
The following example shows the usage of Java HashSet spliterator() method to iterate entries of the HashSet. We've created a HashSet object of Integer. Then few entries are added using add() method and then an spliterator is retrieved using spliterator() method and each value is printed by iterating through the spliterator.
package com.tutorialspoint; import java.util.HashSet; import java.util.Spliterator; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // create an spliterator Spliterator<Integer> spliterator = newset.spliterator(); // check values spliterator.forEachRemaining(v -> System.out.println(v)); } }
Output
Let us compile and run the above program, this will produce the following result.
1 2 3
Getting Spliterator to Iterate Entries of the HashSet of String Example
The following example shows the usage of Java HashSet spliterator() method to iterate entries of the HashSet. We've created a HashSet object of String. Then few entries are added using add() method and then an spliterator is retrieved using spliterator() method and each value is printed by iterating through the spliterator.
package com.tutorialspoint; import java.util.HashSet; import java.util.Spliterator; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // create an spliterator Spliterator<String> spliterator = newset.spliterator(); // check values spliterator.forEachRemaining(v -> System.out.println(v)); } }
Output
Let us compile and run the above program, this will produce the following result.
Learning Easy Simply
Getting Spliterator to Iterate Entries of the HashSet of Objects Example
The following example shows the usage of Java HashSet spliterator() method to iterate entries of the HashSet. We've created a HashSet object of Student objects. Then few entries are added using add() method and then an spliterator is retrieved using spliterator() method and each value is printed by iterating through the spliterator.
package com.tutorialspoint; import java.util.HashSet; import java.util.Spliterator; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // create an spliterator Spliterator<Student> spliterator = newset.spliterator(); // check values spliterator.forEachRemaining(v -> System.out.println(v)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
[ 1, Julie ] [ 2, Robert ] [ 3, Adam ]
To Continue Learning Please Login