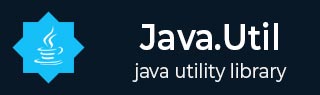
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections synchronizedNavigableMap() Method
Description
The Java Collections synchronizedNavigableMap() method is used to return a synchronized navigable (thread-safe) map backed by the specified navigable map.
Declaration
Following is the declaration for java.util.Collections.synchronizedNavigableMap() method.
public static <K,V> NavigableMap<K,V> synchronizedNavigableMap(NavigableMap<K,V> m)
Parameters
m − This is the navigable map to be "wrapped" in a synchronized navigable map.
Return Value
The method call returns a synchronized navigable view of the specified map.
Exception
NA
Getting Synchronized NavigableMap From a Unsynchronized NavigableMap of String, Integer Pair Example
The following example shows the usage of Java Collection synchronizedNavigableMap(NavigableMap) method. We've created a Map object of String and Integer pairs. Few entries are added and then using synchronizedNavigableMap(NavigableMap) method, we've retrieved the synchronized navigable version of navigable map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.TreeMap; import java.util.NavigableMap; public class CollectionsDemo { public static void main(String[] args) { // create map NavigableMap<String,Integer> map = new TreeMap<String,Integer>(); // populate the map map.put("1",1); map.put("2",2); map.put("3",3); // create a synchronized navigable map NavigableMap<String,Integer> synmap = Collections.synchronizedNavigableMap(map); System.out.println("Synchronized Navigable map is :"+synmap); } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized Navigable map is :{1=1, 2=2, 3=3}
Getting Synchronized NavigableMap From a Unsynchronized NavigableMap of String, String Pair Example
The following example shows the usage of Java Collection synchronizedNavigableMap(NavigableMap) method. We've created a Map object of String and String pairs. Few entries are added and then using synchronizedNavigableMap(NavigableMap) method, we've retrieved the synchronized navigable version of navigable map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.TreeMap; import java.util.NavigableMap; public class CollectionsDemo { public static void main(String[] args) { // create map NavigableMap<String,String> map = new TreeMap<String,String>(); // populate the map map.put("1","TP"); map.put("2","IS"); map.put("3","BEST"); // create a synchronized navigable map NavigableMap<String,String> synmap = Collections.synchronizedNavigableMap(map); System.out.println("Synchronized Navigable map is :"+synmap); } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized Navigable map is :{1=TP, 2=IS, 3=BEST}
Getting Synchronized NavigableMap From a Unsynchronized NavigableMap of String, Object Pair Example
The following example shows the usage of Java Collection synchronizedNavigableMap(Map) method. We've created a Map object of String and Student pairs. Few entries are added and then using synchronizedNavigableMap(Map) method, we've retrieved the synchronized navigable version of map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.TreeMap; import java.util.NavigableMap; public class CollectionsDemo { public static void main(String[] args) { // create map NavigableMap<String,Student> map = new TreeMap<String,Student>(); // populate the map map.put("1",new Student(1, "Julie")); map.put("2",new Student(2, "Robert")); map.put("3",new Student(3, "Adam")); // create a synchronized navigable map NavigableMap<String,Student> synmap = Collections.synchronizedNavigableMap(map); System.out.println("Synchronized Navigable map is :"+synmap); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized Navigable map is :{1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ]}
To Continue Learning Please Login