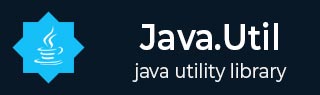
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections synchronizedMap() Method
Description
The Java Collections synchronizedMap() method is used to return a synchronized (thread-safe) map backed by the specified map.
Declaration
Following is the declaration for java.util.Collections.synchronizedMap() method.
public static <K,V> Map<K,V> synchronizedMap(Map<K,V> m)
Parameters
m − This is the map to be "wrapped" in a synchronized map.
Return Value
The method call returns a synchronized view of the specified map.
Exception
NA
Getting Synchronized Map From a Unsynchronized Map of String, Integer Pair Example
The following example shows the usage of Java Collection synchronizedMap(Map) method. We've created a Map object of String and Integer pairs. Few entries are added and then using synchronizedMap(Map) method, we've retrieved the synchronized version of map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.HashMap; import java.util.Map; public class CollectionsDemo { public static void main(String[] args) { // create map Map<String,Integer> map = new HashMap<String,Integer>(); // populate the map map.put("1",1); map.put("2",2); map.put("3",3); // create a synchronized map Map<String,Integer> synmap = Collections.synchronizedMap(map); System.out.println("Synchronized map is :"+synmap); } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized map is :{1=1, 2=2, 3=3}
Getting Synchronized Map From a Unsynchronized Map of String, String Pair Example
The following example shows the usage of Java Collection synchronizedMap(Map) method. We've created a Map object of String and String pairs. Few entries are added and then using synchronizedMap(Map) method, we've retrieved the synchronized version of map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.HashMap; import java.util.Map; public class CollectionsDemo { public static void main(String[] args) { // create map Map<String,String> map = new HashMap<String,String>(); // populate the map map.put("1","TP"); map.put("2","IS"); map.put("3","BEST"); // create a synchronized map Map<String,String> synmap = Collections.synchronizedMap(map); System.out.println("Synchronized map is :"+synmap); } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized map is :{1=TP, 2=IS, 3=BEST}
Getting Synchronized Map From a Unsynchronized Map of String, Object Pair Example
The following example shows the usage of Java Collection synchronizedMap(Map) method. We've created a Map object of String and Student pairs. Few entries are added and then using synchronizedMap(Map) method, we've retrieved the synchronized version of map and printed the map.
package com.tutorialspoint; import java.util.Collections; import java.util.HashMap; import java.util.Map; public class CollectionsDemo { public static void main(String[] args) { // create map Map<String,Student> map = new HashMap<String,Student>(); // populate the map map.put("1",new Student(1, "Julie")); map.put("2",new Student(2, "Robert")); map.put("3",new Student(3, "Adam")); // create a synchronized map Map<String,Student> synmap = Collections.synchronizedMap(map); System.out.println("Synchronized map is :"+synmap); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Synchronized map is :{1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ]}