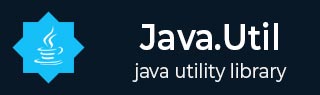
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections emptyNavigableSet() Method
Description
The Java Collections emptyNavigableSet() method is used to get the empty navigable set (immutable). This set is serializable.
Declaration
Following is the declaration for java.util.Collections.emptyNavigableSet() method.
public static final <T> NavigableSet<T> emptyNavigableSet()
Parameters
NA
Return Value
NA
Exception
NA
Getting Empty NavigableSet of Integer Example
The following example shows the usage of Java Collection emptyNavigableSet() method to get an empty navigable set of Integers. We've created an empty navigable set using emptyNavigableSet() method and then tried adding few elements to it which results in exception.
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableSet; public class CollectionsDemo { public static void main(String args[]) { // create an empty navigable set NavigableSet<Integer> emptyNavigableSet = Collections.emptyNavigableSet(); System.out.println("Created empty immutable navigable set: "+emptyNavigableSet); // try to add elements emptyNavigableSet.add(1); emptyNavigableSet.add(2); } }
Output
Let us compile and run the above program, this will produce the following result.Exception will be thrown as the list is immutable.
Created empty immutable navigable set: [] Exception in thread "main" java.lang.UnsupportedOperationException at java.base/java.util.Collections$UnmodifiableCollection.add(Collections.java:1060) at com.tutorialspoint.CollectionsDemo.main(CollectionsDemo.java:15)
Getting Empty NavigableSet of String Example
The following example shows the usage of Java Collection emptyNavigableSet() method to get an empty navigable set of Strings. We've created an empty navigable set using emptyNavigableSet() method and then tried adding few elements to it which results in exception.
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableSet; public class CollectionsDemo { public static void main(String args[]) { // create an empty navigable set NavigableSet<String> emptyNavigableSet = Collections.emptyNavigableSet(); System.out.println("Created empty immutable navigable set: "+emptyNavigableSet); // try to add elements emptyNavigableSet.add("A"); emptyNavigableSet.add("B"); } }
Output
Let us compile and run the above program, this will produce the following result.Exception will be thrown as the list is immutable.
Created empty immutable navigable set: [] Exception in thread "main" java.lang.UnsupportedOperationException at java.base/java.util.Collections$UnmodifiableCollection.add(Collections.java:1060) at com.tutorialspoint.CollectionsDemo.main(CollectionsDemo.java:15)
Getting Empty NavigableSet of Object Example
The following example shows the usage of Java Collection emptyNavigableSet() method to get an empty navigable set of Student objects. We've created an empty navigable set using emptyNavigableSet() method and then tried adding few elements to it which results in exception.
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableSet; public class CollectionsDemo { public static void main(String args[]) { // create an empty navigable set NavigableSet<Student> emptyNavigableSet = Collections.emptyNavigableSet(); System.out.println("Created empty immutable navigable set: "+emptyNavigableSet); // try to add elements emptyNavigableSet.add(new Student(1, "Julie")); emptyNavigableSet.add(new Student(2, "Robert")); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.Exception will be thrown as the list is immutable.
Created empty immutable navigable set: [] Exception in thread "main" java.lang.UnsupportedOperationException at java.base/java.util.Collections$UnmodifiableCollection.add(Collections.java:1060) at com.tutorialspoint.CollectionsDemo.main(CollectionsDemo.java:15)
To Continue Learning Please Login