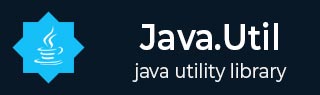
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections disjoint() Method
Description
The Java Collections disjoint(Collection<?>, Collection<?>) method is used to return 'true' if the two specified collections have no elements in common.
Declaration
Following is the declaration for java.util.Collections.disjoint() method.
public static boolean disjoint(Collection<?> c1,Collection<?> c2)
Parameters
c1 − This is a collection.
c2 − This is another collection.
Return Value
NA
Exception
NullPointerException − This is thrown if either collection is null.
Comparing Lists of Integers for Common Values Example
The following example shows the usage of Java Collection disjoint(Collection,Collection) method to compare a list of Integers. We've created two lists with some integers. Using disjoint(Collection,Collection) method, we're comparing content of one list to another for any common element then result is printed.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Integer> srclst = new ArrayList<>(); List<Integer> destlst = new ArrayList<>(); // populate two lists srclst.add(1); srclst.add(2); srclst.add(3); destlst.add(4); destlst.add(5); destlst.add(6); destlst.add(7); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } }
Output
Let us compile and run the above program, this will produce the following result.
No commom elements: true
Comparing Lists of Strings for Common Values Example
The following example shows the usage of Java Collection disjoint(Collection,Collection) method to compare a list of Strings. We've created two lists with some strings. Using disjoint(Collection,Collection) method, we're comparing content of one list to another for any common element then result is printed.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<String> srclst = new ArrayList<>(); List<String> destlst = new ArrayList<>(); // populate two lists srclst.add("A"); srclst.add("B"); srclst.add("C"); destlst.add("D"); destlst.add("E"); destlst.add("F"); destlst.add("G"); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } }
Output
Let us compile and run the above program, this will produce the following result.
No commom elements: true
Comparing Lists of Objects for Common Values Example
The following example shows the usage of Java Collection disjoint(Collection,Collection) method to compare a list of Student objects. We've created two lists with some student objects. Using disjoint(Collection,Collection) method, we're comparing content of one list to another for any common element then result is printed.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Student> srclst = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); List<Student> destlst = new ArrayList<>(Arrays.asList(new Student(4, "Jene"), new Student(5, "Julie"), new Student(6, "Trus"))); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result.
No commom elements: true