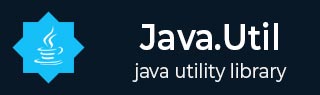
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections checkedSortedSet() Method
Description
The Java Collections checkedSortedSet(SortedSet<E>, Class<E>) method is used to get a dynamically typesafe view of the specified sorted set.
Declaration
Following is the declaration for java.util.Collections.checkedSortedSet() method.
public static <E> SortedSet<E> checkedSortedSet(SortedSet<E> s, Class<E> type)
Parameters
s − This is the sorted set for which a dynamically typesafe view is to be returned.
type −- This is the type of element that s is permitted to hold.
Return Value
The method call returns a dynamically typesafe view of the specified sorted set.
Exception
NA
Getting TypeSafe SortedSet from a SortedSet of Integers Example
The following example shows the usage of Java Collection checkedSortedSet(SortedSet,Class ) method to get a typesafe view of sorted set of integers. We've created a set object with some integers, printed the original set. Using checkedSortedSet(SortedSet, Integer) method, we're getting a sorted set of Integer and then it is printed.
package com.tutorialspoint; import java.util.Collections; import java.util.SortedSet; import java.util.TreeSet; public class CollectionsDemo { public static void main(String[] args) { SortedSet<Integer> set = new TreeSet<>(); set.add(1); set.add(2); set.add(3); set.add(4); set.add(5); System.out.println("Initial collection value: " + set); SortedSet<Integer> safeSet = Collections.checkedSortedSet(set, Integer.class); System.out.println("Typesafe View: "+safeSet); } }
Output
Let us compile and run the above program, this will produce the following result.
Initial collection value: [1, 2, 3, 4, 5] Typesafe View: [1, 2, 3, 4, 5]
Getting TypeSafe SortedSet from a SortedSet of Strings Example
The following example shows the usage of Java Collection checkedSortedSet(SortedSet,Class ) method to get a typesafe view of set of strings. We've created a set object with some strings, printed the original set. Using checkedSortedSet(SortedSet, String) method, we're getting a sorted set of String and then it is printed.
package com.tutorialspoint; import java.util.Collections; import java.util.SortedSet; import java.util.TreeSet; public class CollectionsDemo { public static void main(String[] args) { SortedSet<String> set = new TreeSet<>(); set.add("Welcome"); set.add("to"); set.add("Tutorialspoint"); System.out.println("Initial collection value: " + set); SortedSet<String> safeSet = Collections.checkedSortedSet(set, String.class); System.out.println("Typesafe View: "+safeSet); } }
Output
Let us compile and run the above program, this will produce the following result.
Initial collection value: [Tutorialspoint, Welcome, to] Typesafe View: [Tutorialspoint, Welcome, to]
Getting TypeSafe SortedSet from a SortedSet of Objects Example
The following example shows the usage of Java Collection checkedSortedSet(SortedSet,Class ) method to get a typesafe view of set of Student objects. We've created a SortedSet object with some student objects, printed the original set. Using checkedSortedSet(SortedSet, Student) method, we're getting a SortedSet of Students and then it is printed.
package com.tutorialspoint; import java.util.Collections; import java.util.SortedSet; import java.util.TreeSet; public class CollectionsDemo { public static void main(String[] args) { SortedSet<Student> set = new TreeSet<>(); set.add(new Student(1, "Julie")); set.add(new Student(2, "Robert")); set.add(new Student(3, "Adam")); System.out.println("Initial collection value: " + set); SortedSet<Student> safeSet = Collections.checkedSortedSet(set, Student.class); System.out.println("Typesafe View: "+safeSet); } } class Student implements Comparable<Student> { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public int compareTo(Student student) { return student.rollNo - this.rollNo; } }
Output
Let us compile and run the above program, this will produce the following result.
Initial collection value: [[ 3, Adam ], [ 2, Robert ], [ 1, Julie ]] Typesafe View: [[ 3, Adam ], [ 2, Robert ], [ 1, Julie ]]