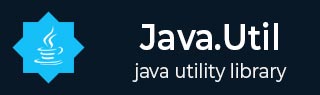
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Calendar isSet() Method
Description
The Java Calendar isSet(int field) method checks if the given calendar field has a value set. Cases that the value has been set by internal fields calculations triggered by a get method call are included.
Declaration
Following is the declaration for java.util.Calendar.isSet method
public final boolean isSet(int field)
Parameters
field − The field to be checked.
Return Value
This method returns true if the given calendar field has a value set; false otherwise.
Exception
NA
Checking Day of Month if Set or Not in a Current Dated Calendar Instance Example
The following example shows the usage of Java Calendar isSet() method. We're creating an instance of a Calendar of current date using getInstance() method and printing the date and time using getTime() method and checking a field is set or not. As next step, we're clearing that field, again print the date and set flag.
package com.tutorialspoint; import java.util.Calendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = Calendar.getInstance(); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); cal.clear(Calendar.DAY_OF_MONTH); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); } }
Output
Let us compile and run the above program, this will produce the following result −
Date And Time Is: Tue Sep 27 10:42:26 IST 2022 Day of month is set: true Date And Time Is: Tue Sep 27 10:42:26 IST 2022 Day of month is set: false
Checking Day of Month if Set or Not in a Current Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar isSet() method. We're creating an instance of a Calendar of current date using GregorianCalendar() method and printing the date and time using getTime() method and checking a field is set or not. As next step, we're clearing that field, again print the date and set flag.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); cal.clear(Calendar.DAY_OF_MONTH); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); } }
Output
Let us compile and run the above program, this will produce the following result −
Date And Time Is: Tue Sep 27 10:43:08 IST 2022 Day of month is set: true Date And Time Is: Tue Sep 27 10:43:08 IST 2022 Day of month is set: false
Checking Day of Month if Set or Not in a Given Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar isSet() method. We're creating an instance of a Calendar of a particular date using GregorianCalendar() method and printing the date and time using getTime() method and checking a field is set or not. As next step, we're clearing that field, again print the date and set flag.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(2025,8,26); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); cal.clear(Calendar.DAY_OF_MONTH); // print the time System.out.println("Date And Time Is: " + cal.getTime()); // determine if the given calendar field has a value set System.out.println("Day of month is set: " + cal.isSet(Calendar.DAY_OF_MONTH)); } }
Output
Let us compile and run the above program, this will produce the following result −
Date And Time Is: Fri Sep 26 00:00:00 IST 2025 Day of month is set: true Date And Time Is: Mon Sep 01 00:00:00 IST 2025 Day of month is set: false