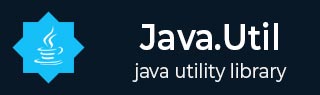
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Calendar getInstance() Method
Description
The Java Calendar getInstance() method gets a calendar using the current time zone and locale.
Declaration
Following is the declaration for java.util.Calendar.getInstance() method
public static Calendar getInstance()
Parameters
NA
Return Value
The method returns a Calendar.
Exception
NA
Java Calendar getInstance(Locale locale) Method
Description
The Java Calendar getInstance(Locale locale) method gets a calendar using the current time zone and specified locale.
Declaration
Following is the declaration for java.util.Calendar.getInstance(Locale locale) method
public static Calendar getInstance(Locale locale)
Parameters
locale − the locale for the calendar data
Return Value
The method returns a Calendar.
Exception
NA
Java Calendar getInstance(TimeZone zone) Method
Description
The Java Calendar getInstance(TimeZone zone) method Gets a calendar using the specified time zone and current locale.
Declaration
Following is the declaration for java.util.Calendar.getInstance(TimeZone zone) method
public static Calendar getInstance(TimeZone zone)
Parameters
zone − the time zone for the calendar data
Return Value
The method returns a Calendar.
Exception
NA
Java Calendar getInstance(TimeZone zone,Locale locale) Method
Description
The Java Calendar getInstance(TimeZone zone,Locale locale) method gets a calendar using the specified time zone and specified locale.
Declaration
Following is the declaration for java.util.Calendar.getInstance(TimeZone zone,Locale locale) method
public static Calendar getInstance(TimeZone zone,Locale locale)
Parameters
zone − the time zone for the calendar data
locale − the locale for the calendar data
Return Value
The method returns a Calendar.
Exception
NA
Getting Current Dated Calendar Instance Example
The following example shows the usage of Java Calendar getInstance() method. We're creating an instance of a Calendar of current date using getInstance() method and printing the date and time.
package com.tutorialspoint; import java.util.Calendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = Calendar.getInstance(); // gets a calendar using the default time zone and locale. System.out.print("Date And Time Is: " + cal.getTime()); } }
Output
Let us compile and run the above program, this will produce the following result −
Date And Time Is: Sat Sep 24 14:20:50 IST 2022
Getting Current Dated Calendar Instance of Given Locale Example
The following example shows the usage of Java Calendar getInstance(Locale) method. We're creating an instance of a Calendar of current date using getInstance(locale) method and printing the date and time.
package com.tutorialspoint; import java.util.Calendar; import java.util.Locale; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = Calendar.getInstance(Locale.CANADA); // print the date for canada System.out.println("Date And Time: " + cal.getTime()); } }
Output
Let us compile and run the above program, this will produce the following result −
Date And Time: Sat Sep 24 14:26:09 IST 2022
Getting Current Dated Calendar Instance of Given TimeZone Example
The following example shows the usage of Java Calendar getInstance(TimeZone) method. We're creating an instance of a Calendar of current date using getInstance(TimeZone) method and printing the time zone of the calendar instance.
package com.tutorialspoint; import java.util.Calendar; import java.util.TimeZone; public class CalendarDemo { public static void main(String[] args) { // create a calendar TimeZone tz1 = TimeZone.getTimeZone("GMT"); Calendar cal1 = Calendar.getInstance(tz1); // create a second calendar with different timezone TimeZone tz2 = TimeZone.getTimeZone("EST"); Calendar cal2 = Calendar.getInstance(tz2); // display time zone for both calendars System.out.println("GMT: " + cal1.getTimeZone().getDisplayName()); System.out.println("EST: " + cal2.getTimeZone().getDisplayName()); } }
Output
Let us compile and run the above program, this will produce the following result −
GMT: Greenwich Mean Time EST: Eastern Standard Time
Getting Current Dated Calendar Instance of Given Locale and TimeZone Example
The following example shows the usage of Java Calendar getInstance(Locale, TimeZone) method. We're creating an instance of a Calendar of current date using getInstance(Locale, TimeZone) method and printing the time zone and locale of the calendar instance.
package com.tutorialspoint; import java.util.Calendar; import java.util.Locale; import java.util.TimeZone; public class CalendarDemo { public static void main(String[] args) { // create a calendar Locale locale1 = Locale.CANADA; TimeZone tz1 = TimeZone.getTimeZone("GMT"); Calendar cal1 = Calendar.getInstance(tz1, locale1); // create a second calendar with different timezone and locale Locale locale2 = Locale.GERMANY; TimeZone tz2 = TimeZone.getTimeZone("EST"); Calendar cal2 = Calendar.getInstance(tz2, locale2); String name1 = locale1.getDisplayName(); String name2 = locale2.getDisplayName(); System.out.println("GMT and Canada: " + cal1.getTimeZone().getDisplayName() + " " + name1); System.out.println("EST and Germany: " + cal2.getTimeZone().getDisplayName() + " " + name2); } }
Output
Let us compile and run the above program, this will produce the following result −
GMT and Canada: Greenwich Mean Time English (Canada) EST and Germany: Eastern Standard Time German (Germany)