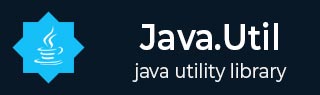
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Arrays compareUnsigned() Method
Description
The Java Arrays compareUnsigned(short[] a, short[] b) method compares the two arrays of shorts lexicographically, numerically treating them unsigned. In case of null arrays comparison, arrays are considered equals and a null array is lexicographically less than a not null array.
Declaration
Following is the declaration for java.util.Arrays.compareUnsigned(short[] a, short[] b) method
public static int compareUnsigned(short[] a, short[] b)
Parameters
a − This is the first array to be compared.
b − This is the second array to be compared.
Return Value
This method returns the value 0 if the first and second array are equal and contain the same elements in the same order; a value less than 0 if the first array is lexicographically less than the second array; and a value greater than 0 if the first array is lexicographically greater than the second array.
Exception
NA
Java Arrays compareUnsigned(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) Method
Description
The Java Arrays compareUnsigned(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) method compares the two arrays of shorts in the given ranges lexicographically, numerically treating them unsigned. In case of any array null, a NullPointerException is thrown.
Declaration
Following is the declaration for java.util.Arrays.compareUnsigned(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) method
public static int compareUnsigned​(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex)
Parameters
a − This is the first array to be compared.
aFromIndex − This is the index of the first element (inclusive) of first array to be compared.
aToIndex − This is the index of the last element (exclusive) of first array to be compared.
b − This is the second array to be compared.
bFromIndex − This is the index of the first element (inclusive) of second array to be compared.
bToIndex − This is the index of the last element (exclusive) of second array to be compared.
Return Value
This method returns the value 0 if, over the specified ranges, the first and second array are equal and contain the same elements in the same order; a value less than 0 if, over the specified ranges, the first array is lexicographically less than the second array; and a value greater than 0 if, over the specified ranges, the first array is lexicographically greater than the second array.
Exception
IllegalArgumentException − if aFromIndex > aToIndex or if bFromIndex > bToIndex
ArrayIndexOutOfBoundsException − if aFromIndex < 0 or aToIndex > a.length or if bFromIndex < 0 or bToIndex > b.length
NullPointerException − if either array is null
Comparing Two Arrays of Same short Values Example
The following example shows the usage of Java Arrays compareUnsigned(short[], short[]) method. First, we've created two arrays of same shorts, and compared them using compareUnsigned() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize first short array short array1[] = { 5, 10, 3, 14, 23 }; // initialize second short array short array2[] = { 5, 10, 3, 14, 23 }; int result = Arrays.compareUnsigned(array1, array2); if(result > 0) { System.out.println("First array is greater than second array."); } else if (result == 0) { System.out.println("Arrays are same."); } else { System.out.println("First array is less than second array."); } } }
Output
Let us compile and run the above program, this will produce the following result −
Arrays are same.
Comparing Two Sub-Arrays of short Values Example
The following example shows the usage of Java Arrays compareUnsigned​(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) method. First, we've created two arrays of different shorts, and compared them using compareUnsigned() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize first short array short array1[] = { 5, 10, 3, 14, 23 }; // initialize second short array short array2[] = { 5, 15, 3, 14, 23 }; int result = Arrays.compareUnsigned(array1, 0, 2, array2, 0, 2); if(result > 0) { System.out.println("First array is greater than second array."); } else if (result == 0) { System.out.println("Arrays are same."); } else { System.out.println("First array is less than second array."); } } }
Output
Let us compile and run the above program, this will produce the following result −
First array is less than second array.
Comparing Two Arrays of Different long Values Example
The following example shows the usage of Java Arrays compareUnsigned(short[], short[]) method. First, we've created two arrays of different shorts, and compared them using compareUnsigned() method. As per the result, the comparison is printed.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize first short array short array1[] = { 5, 15, 3, 14, 23 }; // initialize second short array short array2[] = { 5, 10, 3, 14, 23 }; int result = Arrays.compareUnsigned(array1, array2); if(result > 0) { System.out.println("First array is greater than second array."); } else if (result == 0) { System.out.println("Arrays are same."); } else { System.out.println("First array is less than second array."); } } }
Output
Let us compile and run the above program, this will produce the following result −
First array is greater than second array.
To Continue Learning Please Login