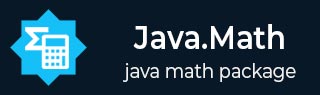
- Java.math package classes
- Java.math - Home
- Java.math - BigDecimal
- Java.math - BigInteger
- Java.math - MathContext
- Java.math package extras
- Java.math - Enumerations
- Java.math - Discussion
Java.math.BigInteger Class
Introduction
The java.math.BigInteger class provides operations analogues to all of Java's primitive integer operators and for all relevant methods from java.lang.Math.
It also provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations. All operations behave as if BigIntegers were represented in two's-complement notation.
Semantics of arithmetic operations and bitwise logical operations are similar to those of Java's integer arithmetic operators and Java's bitwise integer operators respectively. Semantics of shift operations extend those of Java's shift operators to allow for negative shift distances.
Comparison operations perform signed integer comparisons. Modular arithmetic operations are provided to compute residues, perform exponentiation, and compute multiplicative inverses. Bit operations operate on a single bit of the two's-complement representation of their operand.
All methods and constructors in this class throw NullPointerException when passed a null object reference for any input parameter.
Class declaration
Following is the declaration for java.math.BigInteger class −
public class BigInteger extends Number implements Comparable<BigInteger>
Field
Following are the fields for java.math.BigInteger class −
static BigInteger ONE − The BigInteger constant one.
static BigInteger TEN − The BigInteger constant ten.
static BigInteger ZERO − The BigInteger constant zero.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | BigInteger(byte[] val) This constructor is used to translate a byte array containing the two's-complement binary representation of a BigInteger into a BigInteger. |
2 | BigInteger(int signum, byte[] magnitude) This constructor is used to translate the sign-magnitude representation of a BigInteger into a BigInteger. |
3 | BigInteger(int bitLength, int certainty, Random rnd) This constructor is used to construct a randomly generated positive BigInteger that is probably prime, with the specified bitLength. |
4 | BigInteger(int numBits, Random rnd) This constructor is used to construct a randomly generated BigInteger, uniformly distributed over the range 0 to (2numBits - 1), inclusive. |
5 | BigInteger(String val) This constructor is used to translate the decimal String representation of a BigInteger into a BigInteger. |
6 | BigInteger(String val, int radix) This constructor is used to translate the String representation of a BigInteger in the specified radix into a BigInteger. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | BigInteger abs()
This method returns a BigInteger whose value is the absolute value of this BigInteger. |
2 | BigInteger add(BigInteger val)
This method returns a BigInteger whose value is (this + val). |
3 | BigInteger and(BigInteger val)
This method returns a BigInteger whose value is (this & val). |
4 | BigInteger andNot(BigInteger val)
This method returns a BigInteger whose value is (this & ~val). |
5 | int bitCount()
This method returns the number of bits in the two's complement representation of this BigInteger that differ from its sign bit. |
6 | int bitLength()
This method returns the number of bits in the minimal two's-complement representation of this BigInteger, excluding a sign bit. |
7 | BigInteger clearBit(int n)
This method returns a BigInteger whose value is equivalent to this BigInteger with the designated bit cleared. |
8 | int compareTo(BigInteger val)
This method compares this BigInteger with the specified BigInteger. |
9 | BigInteger divide(BigInteger val)
This method returns a BigInteger whose value is (this / val). |
10 | BigInteger[ ] divideAndRemainder(BigInteger val)
This method returns an array of two BigIntegers containing (this / val) followed by (this % val). |
11 | double doubleValue()
This method converts this BigInteger to a double. |
12 | boolean equals(Object x)
This method compares this BigInteger with the specified Object for equality. |
13 | BigInteger flipBit(int n)
This method returns a BigInteger whose value is equivalent to this BigInteger with the designated bit flipped. |
14 | float floatValue()
This method converts this BigInteger to a float. |
15 | BigInteger gcd(BigInteger val)
This method returns a BigInteger whose value is the greatest common divisor of abs(this) and abs(val). |
16 | int getLowestSetBit()
This method returns the index of the rightmost (lowest-order) one bit in this BigInteger (the number of zero bits to the right of the rightmost one bit). |
17 | int hashCode()
This method returns the hash code for this BigInteger. |
18 | int intValue()
This method converts this BigInteger to an int. |
19 | boolean isProbablePrime(int certainty)
This method returns true if this BigInteger is probably prime, false if it's definitely composite. |
20 | long longValue()
This method converts this BigInteger to a long. |
21 | BigInteger max(BigInteger val)
This method returns the maximum of this BigInteger and val. |
22 | BigInteger min(BigInteger val)
This method returns the minimum of this BigInteger and val. |
23 | BigInteger mod(BigInteger m)
This method returns a BigInteger whose value is (this mod m). |
24 | BigInteger modInverse(BigInteger m)
This method returns a BigInteger whose value is (this-1 mod m). |
25 | BigInteger modPow(BigInteger exponent, BigInteger m)
This method returns a BigInteger whose value is (thisexponent mod m). |
26 | BigInteger multiply(BigInteger val)
This method returns a BigInteger whose value is (this * val). |
27 | BigInteger negate()
This method returns a BigInteger whose value is (-this). |
28 | BigInteger nextProbablePrime()
This method returns the first integer greater than this BigInteger that is probably prime. |
29 | BigInteger not()
This method returns a BigInteger whose value is (~this). |
30 | BigInteger or(BigInteger val)
This method returns a BigInteger whose value is (this | val). |
31 | BigInteger pow(int exponent)
This method returns a BigInteger whose value is (thisexponent). |
32 | static BigInteger probablePrime(int bitLength, Random rnd)
This method returns a positive BigInteger that is probably prime, with the specified bitLength. |
33 | BigInteger remainder(BigInteger val)
This method returns a BigInteger whose value is (this % val). |
34 | BigInteger setBit(int n)
This method returns a BigInteger whose value is equivalent to this BigInteger with the designated bit set. |
35 | BigInteger shiftLeft(int n)
This method returns a BigInteger whose value is (this << n). |
36 | BigInteger shiftRight(int n)
This method returns a BigInteger whose value is (this >> n). |
37 | int signum()
This method returns the signum function of this BigInteger. |
38 | BigInteger subtract(BigInteger val)
This method returns a BigInteger whose value is (this - val). |
39 | boolean testBit(int n)
This method returns true if and only if the designated bit is set. |
40 | byte[ ] toByteArray()
This method returns a byte array containing the two's-complement representation of this BigInteger. |
41 | String toString()
This method returns the decimal String representation of this BigInteger. |
42 | String toString(int radix)
This method returns the String representation of this BigInteger in the given radix. |
43 | static BigInteger valueOf(long val)
This method returns a BigInteger whose value is equal to that of the specified long. |
44 | BigInteger xor(BigInteger val)
This method returns a BigInteger whose value is (this ^ val). |