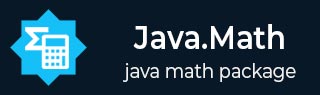
- Java.math package classes
- Java.math - Home
- Java.math - BigDecimal
- Java.math - BigInteger
- Java.math - MathContext
- Java.math package extras
- Java.math - Enumerations
- Java.math - Discussion
Java.math.BigDecimal.add() Method
Description
The java.math.BigDecimal.add(BigDecimal augend, MathContext mc) returns a BigDecimal whose value is (this + augend), with rounding according to the MathContext settings. If either number is zero and the precision setting is nonzero then the other number, rounded if necessary, is used as the result.
Declaration
Following is the declaration for java.math.BigDecimal.add() method.
public BigDecimal add(BigDecimal augend, MathContext mc)
Parameters
augend − Value to be added to this BigDecimal.
mc − The context to use.
Return Value
This method returns a BigDecimal object whose value is this + augend, rounded as necessary
Exception
ArithmeticException − If the result is inexact but the rounding mode is UNNECESSARY.
Example
The following example shows the usage of math.BigDecimal.add() method.
package com.tutorialspoint; import java.math.*; public class BigDecimalDemo { public static void main(String[] args) { // create 3 BigDecimal objects BigDecimal bg1,bg2,bg3,bg4; // assign value to bg1 and bg2 bg1 = new BigDecimal("40.732"); bg2 = new BigDecimal("30.12"); // print bg1 and bg2 value System.out.println("Object Value is " + bg1); System.out.println("Augend value is " + bg2); // create MathContext object with 4 precision MathContext mc = new MathContext(4); // perform add operation on bg1 with augend bg2 and context mc bg3 = bg1.add(bg2,mc); // print bg3 value with System.out.println("Result is " + bg3); } }
Let us compile and run the above program, this will produce the following result −
Object Value is 40.732 Augend value is 30.12 Result is 70.85