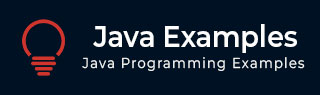
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to iterate through elements of HashMap in Java
Problem Description
How to iterate through elements of HashMap?
Solution
Following example uses iterator Method of Collection class to iterate through the HashMap.
import java.util.*; public class Main { public static void main(String[] args) { HashMap< String, String> hMap = new HashMap< String, String>(); hMap.put("1", "1st"); hMap.put("2", "2nd"); hMap.put("3", "3rd"); Collection cl = hMap.values(); Iterator itr = cl.iterator(); while (itr.hasNext()) { System.out.println(itr.next()); } } }
Result
The above code sample will produce the following result.
1st 2nd 3rd
java_collections.htm
Advertisements