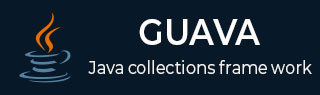
- Guava Tutorial
- Guava - Home
- Guava - Overview
- Guava - Environment Setup
- Guava - Optional Class
- Guava - Preconditions Class
- Guava - Ordering Class
- Guava - Objects Class
- Guava - Range Class
- Guava - Throwables Class
- Guava - Collections Utilities
- Guava - Caching Utilities
- Guava - String Utilities
- Guava - Primitive Utilities
- Guava - Math Utilities
- Guava Useful Resources
- Guava - Quick Guide
- Guava - Useful Resources
- Guava - Discussion
Guava - Splitter Class
Splitter provides various methods to handle splitting operations on string, objects, etc.
Class Declaration
Following is the declaration for com.google.common.base.Splitter class −
@GwtCompatible(emulated = true) public final class Splitter extends Object
Class Methods
Sr.No | Method & Description |
---|---|
1 |
static Splitter fixedLength(int length) Returns a splitter that divides strings into pieces of the given length. |
2 |
Splitter limit(int limit) Returns a splitter that behaves equivalently to this splitter but stops splitting after it reaches the limit. |
3 |
Splitter omitEmptyStrings() Returns a splitter that behaves equivalently to this splitter, but automatically omits empty strings from the results. |
4 |
static Splitter on(char separator) Returns a splitter that uses the given single-character separator. |
5 |
static Splitter on(CharMatcher separatorMatcher) Returns a splitter that considers any single character matched by the given CharMatcher to be a separator. |
6 |
static Splitter on(Pattern separatorPattern) Returns a splitter that considers any subsequence matching pattern to be a separator. |
7 |
static Splitter on(String separator) Returns a splitter that uses the given fixed string as a separator. |
8 |
static Splitter onPattern(String separatorPattern) Returns a splitter that considers any subsequence matching a given pattern (regular expression) to be a separator. |
9 |
Iterable<String> split(CharSequence sequence) Splits sequence into string components and makes them available through an Iterator, which may be lazily evaluated. |
10 |
List<String> splitToList(CharSequence sequence) Splits sequence into string components and returns them as an immutable list. |
11 |
Splitter trimResults() Returns a splitter that behaves equivalently to this splitter, but automatically removes leading and trailing whitespace from each returned substring; equivalent to trimResults(CharMatcher.WHITESPACE). |
12 |
Splitter trimResults(CharMatcher trimmer) Returns a splitter that behaves equivalently to this splitter, but removes all leading or trailing characters matching the given CharMatcher from each returned substring. |
13 |
Splitter.MapSplitter withKeyValueSeparator(char separator) Returns a MapSplitter which splits entries based on this splitter, and splits entries into keys and values using the specified separator. |
14 |
Splitter.MapSplitter withKeyValueSeparator(Splitter keyValueSplitter) Returns a MapSplitter which splits entries based on this splitter, and splits entries into keys and values using the specified key-value splitter. |
15 |
Splitter.MapSplitter withKeyValueSeparator(String separator) Returns a MapSplitter which splits entries based on this splitter, and splits entries into keys and values using the specified separator. |
Methods Inherited
This class inherits methods from the following class −
- java.lang.Object
Example of Splitter Class
Create the following java program using any editor of your choice in say C:/> Guava.
GuavaTester.java
import com.google.common.base.Splitter; public class GuavaTester { public static void main(String args[]) { GuavaTester tester = new GuavaTester(); tester.testSplitter(); } private void testSplitter() { System.out.println(Splitter.on(',') .trimResults() .omitEmptyStrings() .split("the ,quick, ,brown, fox, jumps, over, the, lazy, little dog.")); } }
Verify the Result
Compile the class using javac compiler as follows −
C:\Guava>javac GuavaTester.java
Now run the GuavaTester to see the result.
C:\Guava>java GuavaTester
See the result.
[the, quick, brown, fox, jumps, over, the, lazy, little dog.]