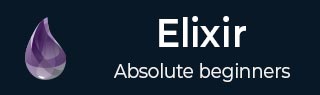
- Elixir Tutorial
- Elixir - Home
- Elixir - Overview
- Elixir - Environment
- Elixir - Basic Syntax
- Elixir - Data Types
- Elixir - Variables
- Elixir - Operators
- Elixir - Pattern Matching
- Elixir - Decision Making
- Elixir - Strings
- Elixir - Char Lists
- Elixir - Lists and Tuples
- Elixir - Keyword Lists
- Elixir - Maps
- Elixir - Modules
- Elixir - Aliases
- Elixir - Functions
- Elixir - Recursion
- Elixir - Loops
- Elixir - Enumerables
- Elixir - Streams
- Elixir - Structs
- Elixir - Protocols
- Elixir - File I/O
- Elixir - Processes
- Elixir - Sigils
- Elixir - Comprehensions
- Elixir - Typespecs
- Elixir - Behaviours
- Elixir - Errors Handling
- Elixir - Macros
- Elixir - Libraries
- Elixir Useful Resources
- Elixir - Quick Guide
- Elixir - Useful Resources
- Elixir - Discussion
Elixir - Streams
Many functions expect an enumerable and return a list back. It means, while performing multiple operations with Enum, each operation is going to generate an intermediate list until we reach the result.
Streams support lazy operations as opposed to eager operations by enums. In short, streams are lazy, composable enumerables. What this means is Streams do not perform an operation unless it is absolutely needed. Let us consider an example to understand this −
odd? = &(rem(&1, 2) != 0) res = 1..100_000 |> Stream.map(&(&1 * 3)) |> Stream.filter(odd?) |> Enum.sum IO.puts(res)
When the above program is run, it produces the following result −
7500000000
In the example given above, 1..100_000 |> Stream.map(&(&1 * 3)) returns a data type, an actual stream, that represents the map computation over the range 1..100_000. It has not yet evaluated this representation. Instead of generating intermediate lists, streams build a series of computations that are invoked only when we pass the underlying stream to the Enum module. Streams are useful when working with large, possibly infinite, collections.
Streams and enums have many functions in common. Streams mainly provide the same functions provided by the Enum module which generated Lists as their return values after performing computations on input enumerables. Some of them are listed in the following table −
Sr.No. | Function and its Description |
---|---|
1 |
chunk(enum, n, step, leftover \\ nil) Streams the enumerable in chunks, containing n items each, where each new chunk starts step elements into the enumerable. |
2 |
concat(enumerables) Creates a stream that enumerates each enumerable in an enumerable. |
3 |
each(enum, fun) Executes the given function for each item. |
4 |
filter(enum, fun) Creates a stream that filters elements according to the given function on enumeration. |
5 |
map(enum, fun) Creates a stream that will apply the given function on enumeration. |
6 |
drop(enum, n) Lazily drops the next n items from the enumerable. |