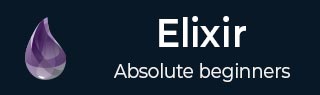
- Elixir Tutorial
- Elixir - Home
- Elixir - Overview
- Elixir - Environment
- Elixir - Basic Syntax
- Elixir - Data Types
- Elixir - Variables
- Elixir - Operators
- Elixir - Pattern Matching
- Elixir - Decision Making
- Elixir - Strings
- Elixir - Char Lists
- Elixir - Lists and Tuples
- Elixir - Keyword Lists
- Elixir - Maps
- Elixir - Modules
- Elixir - Aliases
- Elixir - Functions
- Elixir - Recursion
- Elixir - Loops
- Elixir - Enumerables
- Elixir - Streams
- Elixir - Structs
- Elixir - Protocols
- Elixir - File I/O
- Elixir - Processes
- Elixir - Sigils
- Elixir - Comprehensions
- Elixir - Typespecs
- Elixir - Behaviours
- Elixir - Errors Handling
- Elixir - Macros
- Elixir - Libraries
- Elixir Useful Resources
- Elixir - Quick Guide
- Elixir - Useful Resources
- Elixir - Discussion
Elixir - Misc Operators
Other than the above operators, Elixir also provides a range of other operators that make it quite a powerful language.
Concatenation operator
Elixir provides a string concatenation operator, '<>'. This is used to concatenate 2 strings. For example,
IO.puts("Hello"<>" "<>"world")
The above command generates the following result −
Hello World
Match
The match operator, '=' makes use of the pattern matching feature of the language. We will discuss this operator in detail in a subsequent chapter on Pattern Matching.
Please note that = is not only an assignment operator. When we have the left value as a variable and right value as a literal or another variable, value from right is bound to the variable, i.e., assignment takes place. But if we have a variable on the right and literal on left, pattern matching happens. The same is the case when both values are literals.
Pin
The pin operator, '^' is a unary operator used by prefixing a variable name. It makes sure that the variable when used with the match operator is not assigned a value, but is matched to that value. For example,
a = 12 #assignment a = 13 #assignment ^a = 13 #Pattern matching
Pipe
The pipe operator, '|>' works like the pipe operator in Unix shells. It allows us to pipe the output from one function to the other. For example, if we need to pipe the result of addition in IO.puts, we will use −
(4+3) |> IO.puts
When running above program, it produces following result −
7
This will recognize that we have piped the result of addition in the IO.puts function. This will print 7 on your console.
String Match
The string match operator, '=~', takes a string on the left and either a string or a regular expression on the right. If the string on the right is a substring of left, true is returned. If the regular expression on the right matches the string on the left, true is returned. Otherwise false is returned. For example,
IO.puts("tutorialspoint" =~ "poi") IO.puts("tutorialspoint" =~ ~r/[a-z]*/) IO.puts("tutorialspoint" =~ ~r/[0-9]*/)
When running above program, it produces following result −
true true true
Note that regexes start with a '~r' prefix in Elixir.
Code Point
It is a unary operator, '?' which returns the UTF-8 code point of the character immediately to its right. It can take only one character and accepts Escape Sequences. For example,
IO.puts(?a) IO.puts(?\s)
When running above program, it produces following result −
97 32
Capture
The capture operator, '&' is used when defining anonymous functions. We will discuss this in detail in the functions chapter.
Ternary
Elixir does not have a ternary operator. We can achieve same functionality using the if else statement−
a = if true, do: "True!", else: "False!"
In
This operator checks if left item exists in the enumerable structure on the right. For example, we can check for an atom in a list, tuple, etc. of atoms using this operator −
:yes in [:true, :false, :yes]
The above statement returns true as :yes exists in the list.