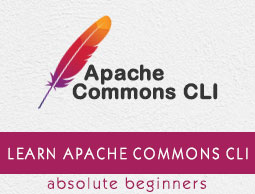
- Apache Commons CLI Tutorial
- Home
- Overview
- Environment Setup
- First Application
- Option Properties
- Boolean Option
- Argument Option
- Properties Option
- Posix Parser
- GNU Parser
- Usage Example
- Help Example
- Apache Commons CLI Resources
- Quick Guide
- Useful Resources
- Discussion
Apache Commons CLI - Argument Option
An Argument option is represented on a command line by its name and its corresponding value. For example, if option is present, then user has to pass its value. Consider the following example, if we are printing logs to some file, for which, we want user to enter name of the log file with the argument option logFile.
Example
CLITester.java
import org.apache.commons.cli.CommandLine; import org.apache.commons.cli.CommandLineParser; import org.apache.commons.cli.DefaultParser; import org.apache.commons.cli.Option; import org.apache.commons.cli.Options; import org.apache.commons.cli.ParseException; public class CLITester { public static void main(String[] args) throws ParseException { Options options = new Options(); Option logfile = Option.builder() .longOpt("logFile") .argName("file" ) .hasArg() .desc("use given file for log" ) .build(); options.addOption(logfile); CommandLineParser parser = new DefaultParser(); CommandLine cmd = parser.parse( options, args); // has the logFile argument been passed? if(cmd.hasOption("logFile")) { //get the logFile argument passed System.out.println( cmd.getOptionValue( "logFile" ) ); } } }
Output
Run the file, while passing --logFile as option, name of the file as value of the option and see the result.
java CLITester --logFile test.log test.log
Advertisements