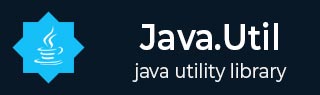
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java BitSet Class
Introduction
The Java BitSet class creates a special type of array that holds bit values. The BitSet array can increase in size as needed. This makes it similar to a vector of bits. This is a legacy class but it has been completely re-engineered in Java 2, version 1.4.
The Java BitSet class implements a vector of bits that grows as needed.Following are the important points about BitSet −
A BitSet is not safe for multithreaded use without external synchronization.
All bits in the set initially have the value false.
Passing a null parameter to any of the methods in a BitSet will result in a NullPointerException.
Class declaration
Following is the declaration for java.util.BitSet class −
public class BitSet extends Object implements Cloneable, Serializable
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | BitSet() This constructor creates a new bit set. |
2 | BitSet(int nbits) This constructor creates a bit set whose initial size is large enough to explicitly represent bits with indices in the range 0 through nbits-1. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | void and(BitSet set)
This method performs a logical AND of this target bit set with the argument bit set. |
2 | void andNot(BitSet set)
This method clears all of the bits in this BitSet whose corresponding bit is set in the specified BitSet. |
3 | int cardinality()
This method returns the number of bits set to true in this BitSet. |
4 | void clear()
This method sets all of the bits in this BitSet to false. |
5 | Object clone()
This method clones this BitSet and produces a new BitSet that is equal to it. |
6 | boolean equals(Object obj)
This method compares this object against the specified object. |
7 | void flip(int bitIndex)
This method sets the bit at the specified index to the complement of its current value. |
8 | boolean get(int bitIndex)
This method returns the value of the bit with the specified index. |
9 | int hashCode()
This method returns the value of the bit with the specified index. |
10 | boolean intersects(BitSet set)
This method returns true if the specified BitSet has any bits set to true that are also set to true in this BitSet. |
11 | boolean isEmpty()
This method returns true if this BitSet contains no bits that are set to true. |
12 | int length()
This method returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. |
13 | int nextClearBit(int fromIndex)
This method returns the index of the first bit that is set to false that occurs on or after the specified starting index. |
14 | int nextSetBit(int fromIndex)
This method returns the index of the first bit that is set to true that occurs on or after the specified starting index. |
15 | void or(BitSet set)
This method performs a logical OR of this bit set with the bit set argument. |
16 | int previousClearBit(int fromIndex)
This method returns the index of the first bit that is set to false that occurs on or before the specified starting index. |
17 | int previousSetBit(int fromIndex)
This method returns the index of the first bit that is set to true that occurs on or after the specified starting index. |
18 | void set(int bitIndex)
This method sets the bit at the specified index to true. |
19 | int size()
This method returns the number of bits of space actually in use by this BitSet to represent bit values. |
20 | IntStream stream()
This method returns a stream of indices for which this BitSet contains a bit in the set state. |
21 | byte[] toByteArray()
This method returns a new bit set containing all the bits in the given byte array. |
22 | long[] toLongArray()
This method returns a new long array containing all the bits in this bit set. |
23 | String toString()
This method returns a string representation of this bit set. |
24 | static BitSet valueOf​(byte[] bytes)
This method returns a new bit set containing all the bits in the given byte array. |
25 | void xor(BitSet set)
This method performs a logical XOR of this bit set with the bit set argument. |
Methods inherited
This class inherits methods from the following classes −
- java.util.Object
Creating a BitSet and Performing Operations on BitSets Example
The following program illustrates several of the methods supported by BitSet data structure −
import java.util.BitSet; public class BitSetDemo { public static void main(String args[]) { BitSet bits1 = new BitSet(16); BitSet bits2 = new BitSet(16); // set some bits for(int i = 0; i < 16; i++) { if((i % 2) == 0) bits1.set(i); if((i % 5) != 0) bits2.set(i); } System.out.println("Initial pattern in bits1: "); System.out.println(bits1); System.out.println("\nInitial pattern in bits2: "); System.out.println(bits2); // AND bits bits2.and(bits1); System.out.println("\nbits2 AND bits1: "); System.out.println(bits2); // OR bits bits2.or(bits1); System.out.println("\nbits2 OR bits1: "); System.out.println(bits2); // XOR bits bits2.xor(bits1); System.out.println("\nbits2 XOR bits1: "); System.out.println(bits2); } }
This will produce the following result −
Output
Initial pattern in bits1: {0, 2, 4, 6, 8, 10, 12, 14} Initial pattern in bits2: {1, 2, 3, 4, 6, 7, 8, 9, 11, 12, 13, 14} bits2 AND bits1: {2, 4, 6, 8, 12, 14} bits2 OR bits1: {0, 2, 4, 6, 8, 10, 12, 14} bits2 XOR bits1: {}