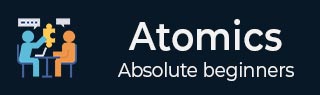
- Atomics Tutorial
- Atomics - Home
- Atomics - Overview
- Atomics Methods
- Atomics - add
- Atomics - and
- Atomics - compareExchange
- Atomics - exchange
- Atomics - isLockFree
- Atomics - load
- Atomics - notify
- Atomics - or
- Atomics - store
- Atomics - sub
- Atomics - xor
- Atomics Useful Resources
- Atomics - Quick Guide
- Atomics - Useful Resources
- Atomics - Discussion
Atomics - Overview
Atomics
The Atomics is an object in JavaScript which provides atomic operations to be performed as static methods. Just like the methods of Math object, the methods and properties of Atomics are also static. Atomics are used with SharedArrayBuffer objects.
The Atomic operations are installed on an Atomics Module. Unlike other global objects, Atomics is not a constructor. Atomics cannot be used with a new operator or can be invoked as a function.
Atomic Operations
Atomic operations are uninterruptible.
When memory is shared, multiple threads can read or write an existed data in the memory. So if any data got changed, there will be a loss of data Atomic operations make sure that predicted values(data) are written and read accurately. Atomic operations wont start until and unless the current operation is finished, so there is no way to change an existed data.
Example
Following is the code demonstrating use of JavaScript Atomics Operation −
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Atomics Example</title> <style> .result { font-size: 20px; border: 1px solid black; } </style> </head> <body onLoad="operate();"> <h1>JavaScript Atomics Properties</h1> <div class="result"></div> <p>Atomics.add(arr, 0, 2)</p> <p>Atomics.load(arr, 0)</p> <script> function operate(){ let container = document.querySelector(".result"); // create a SharedArrayBuffer var buffer = new SharedArrayBuffer(25); var arr = new Uint8Array(buffer); // Initialise element at zeroth position of array with 6 arr[0] = 6; container.innerHTML = Atomics.add(arr, 0, 2) + '<br/>' + Atomics.load(arr, 0); } </script> </body> </html>
Output
Verify the result.