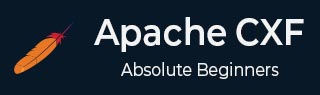
- Apache CXF Tutorial
- Apache CXF - Home
- Apache CXF - Introduction
- Apache CXF with POJO
- Apache CXF with JAX-WS
- Apache CXF with WSDL First
- Apache CXF with JAX-RS
- Apache CXF with JMS
- Apache CXF - Conclusion
- Apache CXF Useful Resources
- Apache CXF - Quick Guide
- Apache CXF - Useful Resources
- Apache CXF - Discussion
Apache CXF with JAX-WS
In this JAX-WS application, we will use Apache CXF-first approach like the earlier POJO application. So first we will create an interface for our web service.
Declaring Service Interface
As in the earlier case, we will create a trivial service that has only one interface method called greetings. The code for the service interface is shown below −
//HelloWorld.java package com.tutorialspoint.cxf.jaxws.helloworld; import javax.jws.WebService; @WebService public interface HelloWorld { String greetings(String text); }
We annotate the interface with a @WebService tag. Next, we will implement this interface.
Implementing Web Interface
The implementation of the web interface is shown here −
//HelloWorldImpl.java package com.tutorialspoint.cxf.jaxws.helloworld; public class HelloWorldImpl implements HelloWorld { @Override public String greetings(String name) { return ("hi " + name); } }
The greetings method is annotated with @Override tag. The method returns a "hi" message to the caller.
Next, we will write the code for developing the server.
Developing Server
Unlike the POJO application, we will now decouple the interface by using the CXF supplied Endpoint class to publish our service. This is done in the following two lines of code −
HelloWorld implementor = new HelloWorldImpl(); Endpoint.publish( "http://localhost:9090/HelloServerPort", implementor, new LoggingFeature() );
The first parameter of the publish method specifies the URL at which our service will be made available to the clients. The second parameter specifies the implementation class for our service. The entire code for the server is shown below −
//Server.java package com.tutorialspoint.cxf.jaxws.helloworld; import javax.xml.ws.Endpoint; import org.apache.cxf.ext.logging.LoggingFeature; public class Server { public static void main(String[] args) throws Exception { HelloWorld implementor = new HelloWorldImpl(); Endpoint.publish("http://localhost:9090/HelloServerPort", implementor, new LoggingFeature()); System.out.println("Server ready..."); Thread.sleep(5 * 60 * 1000); System.out.println("Server exiting ..."); System.exit(0); } }
To deploy our server, you will need to make few more modifications to your project as listed below.
Deploying Server
Finally, to deploy the server application, you will need to make one more modification in pom.xml to setup your application as a web application. The code that you need to add into your pom.xml is given below −
<profiles> <profile> <id>server</id> <build> <defaultGoal>test</defaultGoal> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>exec-maven-plugin</artifactId> <version>1.6.0</version> <executions> <execution> <phase>test</phase> <goals> <goal>java</goal> </goals> <configuration> <mainClass> com.tutorialspoint.cxf.jaxws.helloworld.Server </mainClass> </configuration> </execution> </executions> </plugin> </plugins> </build> </profile> </profiles>
Before you deploy the application, you need to add two more files to your project. These are shown in the screenshot below −
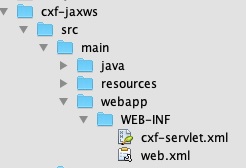
These files are CXF standard files which define the mapping for CXFServlet. The code within the web.xml file is shown here for your quick reference −
//Web.xml <?xml version = "1.0" encoding = "UTF-8"??> <web-app xmlns = "http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" version="2.5" xsi:schemaLocation = "http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"> <display-name>cxf</display-name> <servlet> <description>Apache CXF Endpoint</description> <display-name>cxf</display-name> <servlet-name>cxf</servlet-name> <servlet-class> org.apache.cxf.transport.servlet.CXFServlet </servlet-class> <load-on-startup> 1 </load-on-startup> </servlet> <servlet-mapping> <servlet-name> cxf </servlet-name> <url-pattern> /services/* </url-pattern> </servlet-mapping> <session-config> <session-timeout>60</session-timeout> </session-config> </web-app>
In the cxf-servlet.xml, you declare the properties for your service's endpoint. This is shown in the code snippet below −
<beans ...> <jaxws:endpoint xmlns:helloworld = "https://www.tutorialspoint.com/" id = "helloHTTP" address = "http://localhost:9090/HelloServerPort" serviceName = "helloworld:HelloServiceService" endpointName = "helloworld:HelloServicePort"> </jaxws:endpoint> </beans>
Here we define the id for our service endpoint, the address on which the service will be available, the service name and the endpoint name. Now, you learnt how your service gets routed and processed by a CXF servlet.
The Final pom.xml
The pom.xml includes a few more dependencies. Rather than describing all the dependencies, we have included the final version of pom.xml below −
<?xml version = "1.0" encoding = "UTF-8"??> <project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>cxf-jaxws</artifactId> <version>1.0</version> <packaging>jar</packaging> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <profiles> <profile> <id>server</id> <build> <defaultGoal>test</defaultGoal> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>exec-maven-plugin</artifactId> <version>1.6.0</version> <executions> <execution> <phase>test</phase> <goals> <goal>java</goal> </goals> <configuration> <mainClass> com.tutorialspoint.cxf.jaxws.helloworld.Server </mainClass> </configuration> </execution> </executions> </plugin> </plugins> </build> </profile> <profile> <id>client</id> <build> <defaultGoal>test</defaultGoal> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>exec-maven-plugin</artifactId> <executions> <execution> <phase>test</phase> <goals> <goal>java</goal> <goals> <configuration> <mainClass> com.tutorialspoint.cxf.jaxws.helloworld.Client </mainClass> </configuration> </execution> </executions> </plugin> </plugins> </build> </profile> </profiles> <dependencies> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-frontend-jaxws</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-features-logging</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http-jetty</artifactId> <version>3.3.0</version> </dependency> </dependencies> </project>
Note that it also includes a profile for building client that we will be learning in the later sections of this tutorial.
Running the HelloWorld Service
Now, you are ready to run the web app. In the command window, run the build script using the following command.
mvn clean install
mvn -Pserver
You will see the following message on the console −
INFO: Setting the server's publish address to be http://localhost:9090/HelloServerPort Server ready…
Like earlier, you can test the server by opening the server URL in your browser.
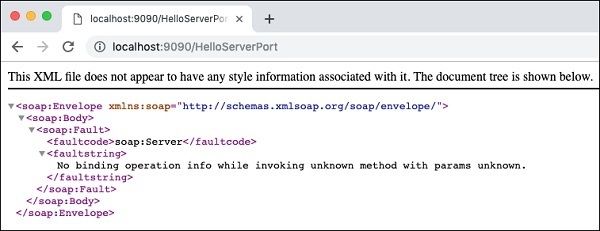
As we did not specify any operation, only a fault message is returned to the browser by our application.
Now, try adding the ?wsdl to your URL and you will see the following output −

So our server application is running as expected. You may use the SOAP Client such as Postman described earlier to further test your service.
In the next section, we will learn how to write a client that uses our service.
Developing Client
Writing the client in a CXF application is as trivial as writing a server. Here is the complete code for the client −
//Client.java package com.tutorialspoint.cxf.jaxws.helloworld; import javax.xml.namespace.QName; import javax.xml.ws.Service; import javax.xml.ws.soap.SOAPBinding; public final class Client { private static final QName SERVICE_NAME = new QName("http://helloworld.jaxws.cxf.tutorialspoint.com/", "HelloWorld"); private static final QName PORT_NAME = new QName("http://helloworld.jaxws.cxf.tutorialspoint.com/", "HelloWorldPort"); private Client() { } public static void main(String[] args) throws Exception { Service service = Service.create(SERVICE_NAME); System.out.println("service created"); String endpointAddress = "http://localhost:9090/HelloServerPort"; service.addPort(PORT_NAME, SOAPBinding.SOAP11HTTP_BINDING, endpointAddress); HelloWorld hw = service.getPort(HelloWorld.class); System.out.println(hw.greetings("World")); } }
Here, we use the CXF supplied Service class to bind to the known service. We call the create method on the Service class to get an instance of the service. We set the known port by calling the addPort method on the service instance.
Now, we are ready to consume the service, which we do by first obtaining the service interface by calling the getPort method on the service instance. Finally, we call our greetings method to print the greetings message on the console.
Now, as you have learned the basics of CXF by using the Apache CXF-First approach, you will now learn how to use CXF with WSDL-First approach in our next chapter.