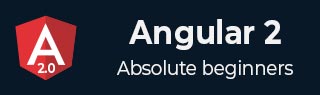
- Angular 2 Tutorial
- Angular 2 - Home
- Angular 2 - Overview
- Angular 2 - Environment
- Angular 2 - Hello World
- Angular 2 - Modules
- Angular 2 - Architecture
- Angular 2 - Components
- Angular 2 - Templates
- Angular 2 - Directives
- Angular 2 - Metadata
- Angular 2 - Data Binding
- CRUD Operations Using HTTP
- Angular 2 - Error Handling
- Angular 2 - Routing
- Angular 2 - Navigation
- Angular 2 - Forms
- Angular 2 - CLI
- Angular 2 - Dependency Injection
- Angular 2 - Advanced Configuration
- Angular 2 - Third Party Controls
- Angular 2 - Data Display
- Angular 2 - Handling Events
- Angular 2 - Transforming Data
- Angular 2 - Custom Pipes
- Angular 2 - User Input
- Angular 2 - Lifecycle Hooks
- Angular 2 - Nested Containers
- Angular 2 - Services
- Angular 2 Useful Resources
- Angular 2 - Questions and Answers
- Angular 2 - Quick Guide
- Angular 2 - Useful Resources
- Angular 2 - Discussion
Angular 2 - Architecture
The following screenshot shows the anatomy of an Angular 2 application. Each application consists of Components. Each component is a logical boundary of functionality for the application. You need to have layered services, which are used to share the functionality across components.
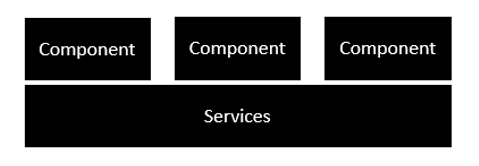
Following is the anatomy of a Component. A component consists of −
Class − This is like a C++ or Java class which consists of properties and methods.
Metadata − This is used to decorate the class and extend the functionality of the class.
Template − This is used to define the HTML view which is displayed in the application.
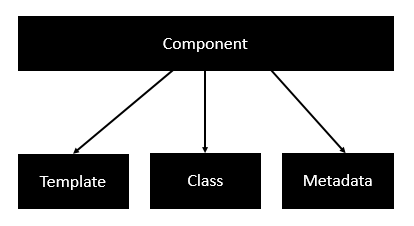
Following is an example of a component.
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { appTitle: string = 'Welcome'; }
Each application is made up of modules. Each Angular 2 application needs to have one Angular Root Module. Each Angular Root module can then have multiple components to separate the functionality.
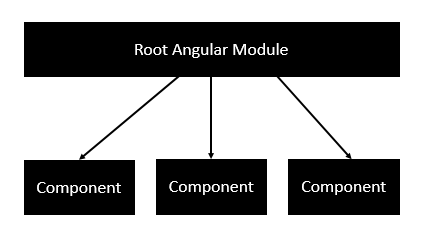
Following is an example of a root module.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; @NgModule ({ imports: [ BrowserModule ], declarations: [ AppComponent ], bootstrap: [ AppComponent ] }) export class AppModule { }
Each application is made up of feature modules where each module has a separate feature of the application. Each Angular feature module can then have multiple components to separate the functionality.
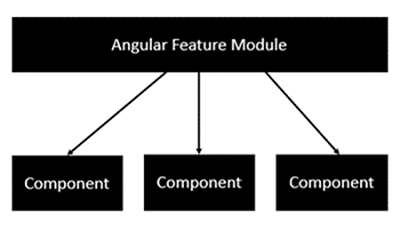