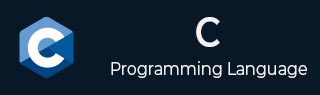
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
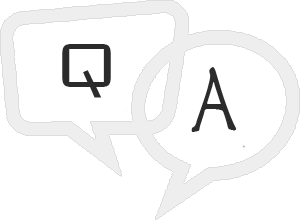
Q 1 - What is the output of the following code snippet?
#include<stdio.h> main() { int *p = 15; printf("%d",*p); }
Answer : C
Explanation
Runtime error, as the pointer variable is not holding proper address, writing/reading the data from the same raises runtime error.
Q 2 - The type name/reserved word ‘short’ is ___
Answer : D
Explanation
short is used as an alternative of short int.
Q 3 - What is the output of the following program?
#include<stdio.h> main() { union abc { int x; char ch; }var; var.ch = 'A'; printf("%d", var.x); }
Answer : C
Explanation
65, as the union variables share common memory for all its elements, x gets ‘A’ whose ASCII value is 65 and is printed.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {10, 20, 30}; printf("%d", *a+1); }
Answer : C
Explanation
*a refers to 10 and adding a 1 to it gives 11.
Q 5 - Which of the following functions disconnects the stream from the file pointer.
Answer : B
Explanation
fclose(), it flushes the buffers associated with the stream and disconnects the stream with the file.
Q 6 - In C programming language, a function prototype is a declaration of the function that just specifies the function’s interface (function's name, argument types and return type) and extracts the body of the function. By defining the function, we get to know what action a particular function is going to perform.
Answer : A
Explanation
Explanation: The function body shall associate a specific task, hence representing the action.
Q 7 - What is a pointer?
A - A keyword used to create variables
B - A variable used to store address of an instruction
Answer : C
Explanation
If var is a variable then &var is an address in memory.
Q 8 - Which of the following statement can be used to free the allocated memory?
Answer : B
Explanation
The library function free() deallocates the memory allocated by calloc(), malloc(), or realloc().
Q 9 - An operation with only one operand is called unary operation.
B - An operation with two operand is called unary operation
C - An operation with unlimited operand is called unary operation
Answer : A
Explanation
Unary operator acts on single expression.
Q 10 - The maximum combined length of the command-line arguments as well as the spaces between adjacent arguments is – a) 120 characters, b) 56 characters, c) Vary from one OS to another
Answer : D
Explanation
The maximum combined length of the command-line arguments and the spaces between adjacent arguments vary from one OS to another.
To Continue Learning Please Login