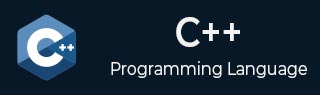
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C++ Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
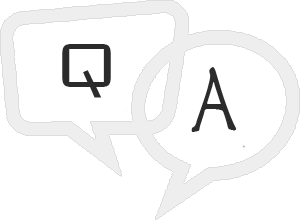
Q 1 - One of the following is true for an inline function.
A - It executes faster as it is treated as a macro internally
B - It executes faster because it priority is more than normal function
C - It doesn’t executes faster compared to a normal function
Answer : A
Explaination
As inline function gets expanded at the line of call like a macro it executes faster.
Q 2 - Which operator is required to be overloaded as member function only?
Answer : D
Explaination
Overloaded assignment operator does the job similar to copy constructor and is required to be overloaded as member function of the class.
Q 3 - Pick up the valid declaration for overloading ++ in postfix form where T is the class name.
Answer : B
Explaination
The parameter int is just to signify that it is the postfix form overloaded. Shouldn’t return reference as per its original behavior.
Q 4 - The pointer which stores always the current active object address is __
Answer : B
Explaination
this is the keyword and acts as a pointer which always holds current active objects.
Q 5 - Which feature of the OOPS gives the concept of reusability?
Answer : C
Explaination
The process of designing a new class (derived) from the existing (base) class to acquire the attributes of the existing is called as inheritance. Inheritance gives the concept of reusability for code/software components.
Q 6 - Which type of data file is analogous to an audio cassette tape?
Answer : B
Explaination
As the access is linear.
Q 7 - What is the output of the following program?
#include <iostream> using namespace std; int main () { // local variable declaration: int x = 1; switch(x) { case 1 : cout << "Hi!" << endl; break; default : cout << "Hello!" << endl; } }
Answer : B
Explaination
Hi, control reaches default-case after comparing the rest of case constants.
#include <iostream> using namespace std; int main () { // local variable declaration: int x = 1; switch(x) { case 1 : cout << "Hi!" << endl; break; default : cout << "Hello!" << endl; } }
Q 8 - What is the output of the following program?
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Answer : B
Explaination
5 3, call by value mechanism can’t alter actual arguments.
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Q 9 - What is the output of the following program?
#include<iostream> using namespace std; void f() { static int i = 3; cout<<i; if(--i) f(); } main() { f(); }
Answer : B
Explaination
As the static variable retains its value from the function calls, the recursion happens thrice.
#include<iostream> using namespace std; void f() { static int i = 3; cout<<i; if(--i) f(); } main() { f(); }
Q 10 - What is the output of the following program?
#include<iostream> using namespace std; main() { char *s = "Fine"; *s = 'N'; cout<<s<<endl; }
Answer : D
Explaination
*s=’N’, trying to change the character at base address to ‘N’ of a constant string leads to runtime error.
#include<iostream> using namespace std; main() { char *s = "Fine"; *s = 'N'; cout<<s<<endl; }
To Continue Learning Please Login