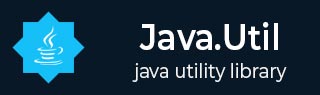
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Properties store() Method
Description
The Java Properties store(OutputStream out,String comments) method writes this property list (key and element pairs) in this Properties table to the output stream in a format suitable for loading into a Properties table using the load(InputStream) method.
Declaration
Following is the declaration for java.util.Properties.store() method
public void store(OutputStream out,String comments)
Parameters
out − an output stream.
comments − a description of the property list.
Return Value
This method returns the previous value of the specified key in this property list, or null if it did not have one.
Exception
IOException − if writing this property list to the specified output stream throws an IOException.
ClassCastException − if this Properties object contains any keys or values that are not Strings.
NullPointerException − if out is null.
Java Properties store(Writer writer,String comments) Method
Description
The java Properties store(Writer writer,String comments) method writes this property list (key and element pairs) in this Properties table to the output character stream in a format suitable for using the load(Reader) method.
Declaration
Following is the declaration for java.util.Properties.store() method
public void store(Writer writer,String comments)
Parameters
out − an output character stream writer.
comments − a description of the property list.
Return Value
This method returns the previous value of the specified key in this property list, or null if it did not have one.
Exception
IOException − if writing this property list to the specified output stream throws an IOException.
ClassCastException − if this Properties object contains any keys or values that are not Strings.
NullPointerException − if out is null.
Storing Entries of Properties to Console Example
The following example shows the usage of Java Properties store(OutputStream, String) method. We've created a Properties object. Then few properties are added and it is printed. Then we've stored the properties into System.out to print on console.
package com.tutorialspoint; import java.io.IOException; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.setProperty("Height", "200"); prop.put("Width", "1500"); // print the list System.out.println("" + prop); try { // store the properties list in an output stream prop.store(System.out, "PropertiesDemo"); } catch (IOException ex) { ex.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
{Height=200, Width=1500} #PropertiesDemo #Mon Dec 26 13:17:45 IST 2022 Height=200 Width=1500
Storing Entries of Properties to a String Example
The following example shows the usage of Java Properties store(OutputStream, String) method. We've created a Properties object. Then few properties are added and it is printed. Then we've stored the properties into System.out to print on console.
package com.tutorialspoint; import java.io.IOException; import java.io.StringWriter; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); StringWriter sw = new StringWriter(); // add some properties prop.setProperty("Height", "200"); prop.put("Width", "1500"); // print the list System.out.println("" + prop); try { // store the properties list in an output writer prop.store(sw, "PropertiesDemo"); // print the writer System.out.println("" + sw.toString()); } catch (IOException ex) { ex.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
{Height=200, Width=1500} #PropertiesDemo #Mon Dec 26 13:19:47 IST 2022 Height=200 Width=1500
To Continue Learning Please Login