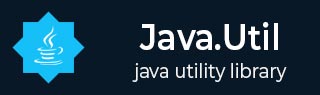
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TimerTask Class
Introduction
The Java TimerTask class represents a task that can be scheduled for one-time or repeated execution by a Timer.
Class declaration
Following is the declaration for java.util.TimerTask class −
public abstract class TimerTask extends Object implements Runnable
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | protected TimerTask() This constructor creates a new timer task. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean cancel()
This method cancels this timer task. |
2 | abstract void run()
This method represents the action to be performed by this timer task. |
3 | long scheduledExecutionTime()
This method returns the scheduled execution time of the most recent actual execution of this task. |
Methods inherited
This class inherits methods from the following classes −
- java.util.Object
Running a Timer Task for a given Schedule Example
The following example shows the usage of Java Timer run() method to run the timer operation as per schedule. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerTaskDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); try { Thread.sleep(500); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
working on working on working on working on working on working on
To Continue Learning Please Login