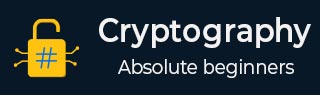
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Decryption Transposition Cipher
In the last chapter we learned about transposition cipher encryption. Now we will see decryption of transposition ciphers and its implementations using different methods.
The decryption process simply involves reversing the steps of the encryption algorithm. We use the same key to find the correct order of reading the columns to recover the actual message.
Algorithm for Transposition Cipher Decryption
Below is the algorithm for Transposition Cipher decryption −
Inputs
Ciphertext − The encrypted message.
Key − The same key used for encryption.
Steps
First we need to divide the ciphertext into rows as per the key length. Means we will create one row for each character in the key.
Now we will create an empty grid with the same number of rows as the key length and a number of columns equal to the length of the ciphertext divided by the key length.
Next we have to fill the grid as per the key. So iterate over the ciphertext characters to determine its column index.
And add the character to the respective cell in the grid.
Start with the first column and read characters downwards, moving to the next column once you reach the bottom. Continue this process for all columns. This rearranges the characters as per the original transposition order.
Print the plaintext message.
Example
Inputs
Ciphertext: OLHEL LWRDO
Key: SECRET
For example, if we know the key that is "SECRET" and the original message was transposed as per the order of the letters in the key. We can rearrange the columns like below −
Key: | S E C R E T |
Columns: | 5, 1, 2, 4, 6, 3 |
Now, we will reorder the columns of the transposed message "OLHEL LWRDO" as per the key like this −
S: | O | L |
E: | H | E |
C: | L | W |
R: | H | R |
E: | E | D |
T: | L | O |
Finally, we read the characters row by row to get the original message −
Original Message: HELLO WORLD
So, the decrypted message for the encrypted message "OLHEL LWRDO" using the key "SECRET" is "HELLO WORLD".
Yay! We have decrypted our message using a transposition cipher.
Implementation using Python
The decryption for transposition cipher can be implemented using different methods −
Using Math Module
Using Pyperclip module
So let us see these two methods one by one in the following sections −
Using Math Module
This Python code decrypts a message that has been encrypted using a transposition cipher. And we will use a math module to perform mathematical operations mainly to calculate the number of columns needed to decrypt the message. And math.ceil() is used to round up the result of dividing the length of the message by the key. So this code efficiently decrypts transposition cipher messages.
Example
Below is a Python code for the transposition cipher decryption algorithm using the Math module. See the program below −
import math def transposition_decrypt(key, message): num_of_columns = math.ceil(len(message) / key) num_of_rows = key num_of_shaded_boxes = (num_of_columns * num_of_rows) - len(message) plaintext = [''] * num_of_columns col = 0 row = 0 for symbol in message: plaintext[col] += symbol col += 1 if (col == num_of_columns) or (col == num_of_columns - 1 and row >= num_of_rows - num_of_shaded_boxes): col = 0 row += 1 return ''.join(plaintext) ciphertext = 'Toners raiCntisippoh' key = 6 plaintext = transposition_decrypt(key, ciphertext) print("Cipher Text: ", ciphertext) print("The plain text is: ", plaintext)
Following is the output of the above example −
Input/Output
Cipher Text: Toners raiCntisippoh The plain text is: Transposition Cipher
In the above output you can see that the ciphertext message was Toners raiCntisippoh and the decrypted message is Transposition Cipher.
Using Pyperclip module
In this example we will use the pyperclip module of Python and it is used to copy and paste clipboard functions. So we will use this module to copy our decrypted message on the clipboard.
This code is similar to the above code but in this code we are using pyperclip module to copy the decrypted message to the clipboard.
Example
Below is a Python code for transposition cipher decryption algorithm using pyperclip module. Please check the code below −
import math import pyperclip def transposition_decrypt(key, message): num_of_columns = math.ceil(len(message) / key) num_of_rows = key num_of_shaded_boxes = (num_of_columns * num_of_rows) - len(message) plaintext = [''] * num_of_columns col = 0 row = 0 for symbol in message: plaintext[col] += symbol col += 1 if (col == num_of_columns) or (col == num_of_columns - 1 and row >= num_of_rows - num_of_shaded_boxes): col = 0 row += 1 return ''.join(plaintext) ciphertext = 'Toners raiCntisippoh' key = 6 plaintext = transposition_decrypt(key, ciphertext) print("Cipher Text:", ciphertext) print("The plain text is:", plaintext) # Copy the decrypted plaintext to the clipboard pyperclip.copy(plaintext) print("The Decrypted Message is Copied to the Clipboard")
Following is the output of the above example −
Input/Output
Cipher Text: Toners raiCntisippoh The plain text is: Transposition Cipher The Decrypted Message is Copied to the Clipboard
In the above output we can see that the plain text is copied to the clipboard and there is a message seen that "The Decrypted Message is Copied to the Clipboard".
Implementation using Java
In this we are going to use Java programming langugage to implement the decryption of transposition cipher. Basically we will reverse the process of encryption in this implementation using Java.
See the code below in Java −
Example
public class TranspositionCipher { // Function to decrypt using transposition cipher public static String decrypt(String ciphertext, int key) { StringBuilder plaintext = new StringBuilder(); int cols = (int) Math.ceil((double) ciphertext.length() / key); char[][] matrix = new char[cols][key]; // Fill matrix with ciphertext characters int index = 0; for (int j = 0; j < key; ++j) { for (int i = 0; i < cols; ++i) { if (index < ciphertext.length()) matrix[i][j] = ciphertext.charAt(index++); else matrix[i][j] = ' '; } } // Read matrix row-wise to get plaintext for (int i = 0; i < cols; ++i) { for (int j = 0; j < key; ++j) { plaintext.append(matrix[i][j]); } } return plaintext.toString(); } public static void main(String[] args) { String ciphertext = "Hohiebtlre,isrei ll s yafwdlT v uuo "; int key = 4; System.out.println("The Encrypted text: " + ciphertext); String decryptedText = decrypt(ciphertext, key); System.out.println("Decrypted text: " + decryptedText); } }
Following is the output of the above example −
Input/Output
The Encrypted text: Hohiebtlre,isrei ll s yafwdlT v uuo Decrypted text: Hello, This is very beautiful world
Implementation using C++
Now we will use C++ programming langugage to implement the decryption algorithm for transposition cipher. In which we will reverse the encryption process by the decrypt function. It finds the required number of columns by calculating the length of the ciphertext and the key. It adds the characters from the ciphertext column-wise into a matrix. After that, it builds the plaintext using row-by-row reading of the matrix.
Following is the implementation of decryption transposition cipher using C++ −
Example
#include <iostream> #include <string> #include <cmath> // Function to decrypt using transposition cipher std::string decrypt(std::string ciphertext, int key) { std::string plaintext = ""; int cols = (ciphertext.length() + key - 1) / key; char matrix[cols][key]; // Fill matrix with ciphertext characters int index = 0; for (int j = 0; j < key; ++j) { for (int i = 0; i < cols; ++i) { if (index < ciphertext.length()) matrix[i][j] = ciphertext[index++]; else matrix[i][j] = ' '; } } // Read matrix row-wise to get plaintext for (int i = 0; i < cols; ++i) { for (int j = 0; j < key; ++j) { plaintext += matrix[i][j]; } } return plaintext; } int main() { std::string ciphertext = "Houiptme,tao il oliFllTrsnay"; int key = 4; std::cout << "The Encrypted text: " << ciphertext << std::endl; std::string decrypted_text = decrypt(ciphertext, key); std::cout << "The Decrypted text: " << decrypted_text << std::endl; return 0; }
Following is the output of the above example −
Input/Output
The Encrypted text: Houiptme,tao il oliFllTrsnay The Decrypted text: Hello, Tutorialspoint Family
Summary
In this chapter, we have seen how we can decipher the ciphertext back to its original form using the Transposition Cipher decryption algorithm in Python, C++ and Java.
To Continue Learning Please Login