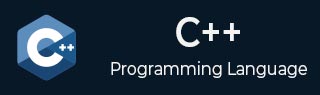
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C++ Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
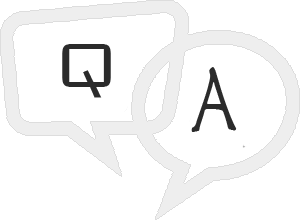
Q 1 - What is the output of the following program?
#include<iostream> using namespace std; class abc { void f(); void g(); int x; }; main() { cout<<sizeof(abc)<<endl; }
Answer : B
Explaination
Only the class member variables constitutes as the size of the class or its object.
#include<iostream> using namespace std; class abc { void f(); void g(); int x; }; main() { cout<<sizeof(abc)<<endl; }
Q 2 - The operator used to access member function of a structure using its object.
Answer : A
Explaination
Just the way we use dot (.) operator to access members of the class, in similar it is used to access the members of the structure too.
Q 3 - In the following program f() is overloaded.
void f(int x) { } int f(signed x) { return 1; } main() { }
Answer : B
Explaination
No, as both the functions arguments is same and compiler ignores return type to consider overloading though different in return type.
Q 4 - How can we make an class act as an interface in C++?
A - By only providing all the functions as virtual functions in the class.
B - Defining the class following with the keyword virtual
Answer : A
Explaination
There are no keywords in C++ such as abstract and interface.
Q 5 - What is the full form of RTTI.
A - Runtime type identification
B - Runtime template identification
Answer : A
Explaination
Q 6 - With respective to streams >> (operator) is called as
Answer : B
Explaination
It extracts the data from stream into variables.
Q 7 - What is the output of the following program?
#include<iostream> using namespace std; main() { int x = 5; if(x==5) { if(x==5) break; cout<<"Hello"; } cout<<”Hi”; }
Answer : A
Explaination
compile error, keyword break can appear only within loop/switch statement.
#include<iostream> using namespace std; main() { int x = 5; if(x==5) { if(x==5) break; cout<<"Hello"; } cout<<”Hi”; }
Q 8 - What is the size of the following union definition?
#include<iostream> using namespace std; main() { union abc { char a, b, c, d, e, f, g, h; int i; }; cout<<sizeof(abc); }
Answer : C
Explaination
union size is biggest element size of it. All the elements of the union share common memory.
#include<iostream> using namespace std; main() { union abc { char a, b, c, d, e, f, g, h; int i; }; cout<<sizeof(abc); }
Answer : A
Explaination
As the code of inline function gets expanded at the line of call, therefore it gets executed faster with no overhead of context switch
Q 10 - Does both the loops in the following programs prints the correct string length?
#include<iostream> using namespace std; main() { int i; char s[] = "hello"; for(i=0; s[i]; ++i); cout<<i<<endl; i=0; while(s[i++]); cout<<i; }
A - Yes, both the loops prints the correct length
B - Only for loop prints the correct length
Answer : B
Explaination
In while loop 'i' gets incremented after checking for '\0', hence giving 1 more than the length.
#include<iostream> using namespace std; main() { int i; char s[] = "hello"; for(i=0; s[i]; ++i); cout<<i<<endl; i=0; while(s[i++]); cout<<i; }
To Continue Learning Please Login