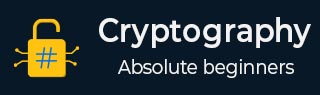
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Implementation of One Time Pad Cipher
In the previous chapter we saw what is One−Time Pad cipher, How it works and advantages and drawback of it. So now in this chapter we will see the implementation of One−Time Pad cipher using Python, Java and C++.
Implementation in Python
This problem can be solved using different methods like −
Using modulo operation
Using XOR operation
Using Python's onetimepad module
Using random, string and sqlite3 modules
So we will discuss each of these methods in depth in the sections below.
Using modulo operation
In the code, the modulo operator (%) makes sure that the resulting letter stays within the range of English uppercase letters (ASCII values from 65 to 90). Here's a breakdown of how it's used in encryption and decryption:
Encryption − For each character in the message and the corresponding character in the key, their ASCII values are added. The sum is then divided by 26 and the remainder is kept (using modulo). This ensures it falls within the range 0 to 25. 65 is added to this value to bring it back to the range of ASCII values for uppercase letters (65 to 90). Finally, the resulting ASCII value is converted back to its corresponding character using the chr() function.
Decryption − Similar to encryption, the ASCII values of corresponding message and key characters are added. Again, modulo 26 is used to ensure the sum falls within the range 0 to 25. To decrypt, 26 is subtracted from this sum and the result is used to get the corresponding character using chr().
Example
Below is the Implementation in python using modulo operation for one time pad cipher.
def otp_encrypt(message, key): encrypted_message = "" for i in range(len(message)): char = chr((ord(message[i]) + ord(key[i])) % 26 + 65) encrypted_message += char return encrypted_message def otp_decrypt(encrypted_message, key): decrypted_message = "" for i in range(len(encrypted_message)): char = chr((ord(encrypted_message[i]) - ord(key[i])) % 26 + 65) decrypted_message += char return decrypted_message # plaintext and key message = "HELLO" key = "WORLD" encrypted_message = otp_encrypt(message, key) print("Encrypted message:", encrypted_message) decrypted_message = otp_decrypt(encrypted_message, key) print("Decrypted message:", decrypted_message)
Following is the output of the above example −
Input/Output
Encrypted message: DSCWR Decrypted message: HELLO
Using XOR operation
Using the XOR operation, the code makes sure that encrypted characters are created and decrypted in a way that makes it very hard to get the original message or key from just the encrypted text. XOR, which stands for "exclusive or," is a bitwise operation that uses the bits of its inputs to get a result. When you use XOR twice with the same value, you get back the original value. This makes XOR a good choice for encryption in the One-Time Pad method.
Example
Check the python program below for one time pad cipher using XOR operation −
def otp_encrypt(message, key): encrypted_message = "" for i in range(len(message)): char = chr(ord(message[i]) ^ ord(key[i])) encrypted_message += char return encrypted_message def otp_decrypt(encrypted_message, key): decrypted_message = "" for i in range(len(encrypted_message)): char = chr(ord(encrypted_message[i]) ^ ord(key[i])) decrypted_message += char return decrypted_message # plaintext and key message = "HELLO" key = "WORLD" encrypted_message = otp_encrypt(message, key) print("Encrypted message:", encrypted_message) decrypted_message = otp_decrypt(encrypted_message, key) print("Decrypted message:", decrypted_message)
Following is the output of the above example −
Input/Output
Encrypted message: DSCWR Decrypted message: HELLO
Using Python's onetimepad module
To encrypt and decrypt messages using the One−Time Pad method, we utilize the onetimepad module. It provides functions for both encryption and decryption. This module streamlines the process by offering ready-to-use functions for One−Time Pad operations. With just a few lines of code, you can effortlessly encrypt and decrypt messages.
Example
Check the below code for one time pad cipher using Python's onetimepad module −
import onetimepad def otp_encrypt(message, key): encrypted_message = onetimepad.encrypt(message, key) return encrypted_message def otp_decrypt(encrypted_message, key): decrypted_message = onetimepad.decrypt(encrypted_message, key) return decrypted_message # plain text and key message = "This is a secret message" key = "My key" encrypted_message = otp_encrypt(message, key) print("Encrypted message:", encrypted_message) decrypted_message = otp_decrypt(encrypted_message, key) print("Decrypted message:", decrypted_message)
Following is the output of the above example −
Input/Output
Encrypted message: 1911491845103e59414b161c2e0b451f4514280a530a021c Decrypted message: This is a secret message
Using random, string and sqlite3 modules
This Python code implements a simple command-line tool for creating and managing encrypted messages using a one time pad cipher technique. This code sets up the basics for working with encrypted messages. It uses a one time pad cipher technique, and it shows how to generate keys, encrypt messages, and decrypt them.
In this Implementation we will be using random, string and sqlite3 modules. These modules are versatile and widely used in Python programming for tasks ranging from basic string manipulation to database management and random data generation.
Example
Check the Python program below −
import random import string import sqlite3 PUNC = string.punctuation ALPHABET = string.ascii_letters def initialize(): connection = sqlite3.connect(":memory:", isolation_level=None, check_same_thread=False) cursor = connection.cursor() cursor.execute( "CREATE TABLE used_keys (" "id INTEGER PRIMARY KEY AUTOINCREMENT," "key TEXT" ")" ) return cursor def create_key(_string, db_cursor): """ Create the key from a provided string """ retval = "" set_string = "" used_keys = db_cursor.execute("SELECT key FROM used_keys").fetchall() id_number = len(used_keys) + 1 for c in _string: if c in PUNC or c.isspace(): continue set_string += c key_length = len(set_string) acceptable_key_characters = string.ascii_letters for _ in range(key_length): retval += random.choice(acceptable_key_characters) if retval not in [key[0] for key in used_keys]: db_cursor.execute("INSERT INTO used_keys(id, key) VALUES (?, ?)", (id_number, retval)) return retval, set_string else: return create_key(_string, db_cursor) def encode_cipher(_string, key): """ Encode the string using a generated unique key """ retval = "" for k, v in zip(_string, key): c_index = ALPHABET.index(k) key_index = ALPHABET.index(v) cipher_index = (c_index + key_index) % 52 retval += ALPHABET[cipher_index] return retval def decode_cipher(encoded, key): # Decode the encoded string retval = "" for k, v in zip(encoded, key): c_index = ALPHABET.index(k) key_index = ALPHABET.index(v) decode = (c_index - key_index) % 52 retval += ALPHABET[decode] return retval def main(): # Main function exited = False choices = {"1": "Show keys", "2": "Create new key", "3": "Decode a cipher", "4": "Exit"} cursor = initialize() separator = "-" * 35 print("Database initialized, what would you like to do:") try: while not exited: for item in sorted(choices.keys()): print("[{}] {}".format(item, choices[item])) choice = input(">> ") if choice == "1": keys = cursor.execute("SELECT key FROM used_keys") print(separator) for key in keys.fetchall(): print(key[0]) print(separator) elif choice == "2": phrase = input("Enter your secret phrase: ") key, set_string = create_key(phrase, cursor) encoded = encode_cipher(set_string, key) print(separator) print("Encoded message: '{}'".format(encoded)) print(separator) elif choice == "3": encoded_cipher = input("Enter an encoded cipher: ") encode_key = input("Enter the cipher key: ") decoded = decode_cipher(encoded_cipher, encode_key) print(separator) print("Decoded message: '{}'".format(decoded)) print(separator) elif choice == "4": print("Database destroyed") exited = True except KeyboardInterrupt: print("Database has been destroyed") if __name__ == "__main__": main()
Following is the output of the above example −
Input/Output

Implementation using Java
Now we are going to implenent one time pad cipher with the help of Java. In which we will create a class for OTP and inside the class we will have three functions one for encrypting and message, Second one for decrypting the message and third one will be the main method.
In the encryptMessage() function we will have two parameters: plaintext and secret key. So we will use a StringBuilder to initialize a storage for ciphertext.
After calculating the length of the key. We will iterate over each plaintext character. And then we will add the respective key character and applies modulo 128 for ASCII range. After that we will append encrypted character to the ciphertext StringBuilder. And in the decryptMessage() function reverses the process of encryptMessage() function.
Example
Following is the implementation of One Time Pad using Java −
public class OneTimePad { // Encryption function to encrypt the given message public static String encryptMessage(String plaintext, String key) { StringBuilder ciphertext = new StringBuilder(); int keyLength = key.length(); for (int i = 0; i < plaintext.length(); i++) { char encryptedChar = (char) ((plaintext.charAt(i) + key.charAt(i % keyLength)) % 128); ciphertext.append(encryptedChar); } return ciphertext.toString(); } // Decryption function to decrypt the message public static String decryptMessage(String ciphertext, String key) { StringBuilder plaintext = new StringBuilder(); int keyLength = key.length(); for (int i = 0; i < ciphertext.length(); i++) { char decryptedChar = (char) ((ciphertext.charAt(i) - key.charAt(i % keyLength) + 128) % 128); plaintext.append(decryptedChar); } return plaintext.toString(); } public static void main(String[] args) { String plaintext = "Welcome to our Website"; String key = "Secret"; // Repeat the key if it's shorter than the plaintext while (key.length() < plaintext.length()) { key += key; } // Encryption String et = encryptMessage(plaintext, key); System.out.println("The Encrypted Text: " + et); // Decryption String dt = decryptMessage(et, key); System.out.println("The Decrypted Text: " + dt); } }
Following is the output of the above example −
Input/Output
The Encrypted Text: *JOUTa8 Wa cHW IJVFNWW The Decrypted Text: Welcome to our Website
Implementation using C++
Now we will implement One− Pad cipher with the help of C++ programming langugage. This code showcases the encryption and decryption of messages using the One-Time Pad cipher in C++. Two distinct functions, one for encryption and one for decryption, are defined and utilized along with a main function that illustrates their application.
Example
Below is the implementation using c++ for One time Pad Cipher −
#include <iostream> #include <string> using namespace std; // Encryption function to encrypt the message string encryptMessage(string plaintext, string key) { string ciphertext = ""; for (int i = 0; i < plaintext.length(); i++) { char encryptedChar = (plaintext[i] + key[i]) % 128; ciphertext += encryptedChar; } return ciphertext; } // Decryption function to decrypt the message string decryptMessage(string ciphertext, string key) { string plaintext = ""; for (int i = 0; i < ciphertext.length(); i++) { char decryptedChar = (ciphertext[i] - key[i] + 128) % 128; plaintext += decryptedChar; } return plaintext; } int main() { string plaintext = "Hello This is Tutorialspoint"; string key = "Secret"; // Encryption string et = encryptMessage(plaintext, key); cout << "The Encrypted Text: " << et << endl; // Decryption string dt = decryptMessage(et, key); cout << "The Decrypted Text: " << dt << endl; return 0; }
Following is the output of the above example −
Input/Output
The Encrypted Text: O^T Th!F=qr TuTmlialsp int The Decrypted Text: Hello Th|}qr Tutorialspoint
To Continue Learning Please Login