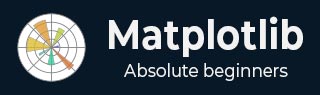
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Arrows
What are Arrows in Matplotlib?
In Matplotlib library Arrows refer to the graphical elements used to indicate direction, connection between points and to highlight specific features within plots. Arrows can be added to plots using the plt.arrow() function or incorporated within annotations by using the plt.annotate() function.
Arrows in Matplotlib library are versatile elements used to visually depict directionality, connections or highlights within the plots for aiding in better communication of information in visualizations. We can adjust parameters like coordinates, lengths, colors and styles to suit the specific visualization needs.
The plt.arrow() function in matplotlib library creates arrows between two points on a plot.
Syntax
The following is the syntax and parameters of the plt.arrow() function.
plt.arrow(x, y, dx, dy, kwargs)
Where,
x, y − These are the starting point coordinates of the arrow.
dx, dy − These are lengths of the arrow in the x and y directions.
kwargs − We can add additional keyword arguments to customize arrow properties such as color, width, style etc.
Adding arrows to the line plot
In this example we are plotting a plot by creating the arrows between the given two points on the plot by using the plt.arrow() function. To this function we passed the x, y, dx and dy points as input parameters for creating arrows in those mentioned points. In addition we passed the parameters such as length, width and color of the arrow.
Example
import matplotlib.pyplot as plt # Creating a line plot plt.plot([0, 1], [0, 1]) # Adding an arrow plt.arrow(0.2, 0.2, 0.4, 0.4, head_width=0.05, head_length=0.1, fc='red', ec='blue') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Arrow created by using plt.arrow() function') # show grid of the plot plt.grid(True) plt.show()
Output

Adding arrow separately
Here this is another example of creating the arrows. As we know arrows can also be integrated into annotations using plt.annotate() by specifying arrowprops to define arrow styles. In this example 'Arrow Annotation' is connected to point (0.5, 0.5) with an arrow style specified by arrowprops.
Example
import matplotlib.pyplot as plt # Creating a plot plt.plot([0, 1], [0, 1]) # Adding an arrow with annotation plt.annotate('Arrow Annotation', xy=(0.5, 0.5), xytext=(0.2, 0.2), arrowprops=dict(facecolor='yellow',ec = 'red', arrowstyle='->')) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Arrow Annotation Example') # Displaying the grid plt.grid(True) plt.show()
Output

Plotting distance arrows in technical drawing
To plot the distance arrows in technical drawing in matplotlib we can use annotate() method with arrow properties.
Example
from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True plt.axhline(3.5) plt.axhline(2.5) plt.annotate( '', xy=(0.5, 3.5), xycoords='data', xytext=(0.5, 2.5), textcoords='data', arrowprops={'arrowstyle': '<->'}) plt.annotate( '$\it{d=1}$', xy=(0.501, 3.0), xycoords='data', xytext=(0.5, 3.5), textcoords='offset points') plt.show()
Output

To Continue Learning Please Login