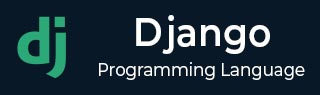
- Django Basic Concepts
- Django - Home
- Django - Basics
- Django - Overview
- Django - Environment
- Django - Creating a Project
- Django - Apps Life Cycle
- Django - Creating Views
- Django - URL Mapping
- Django - Templates System
- Django - MVT
- Django Admin
- Django Admin - Interface
- Django Admin - Create User
- Django Admin - Include Models
- Django Admin - Set Fields to Display
- Django Admin - Update Objects
- Django Models
- Django - Models
- Django - Insert Data
- Django - Update Data
- Django - Delete Data
- Django - Update Model
- Django Advanced
- Django - Page Redirection
- Django - Sending E-mails
- Django - Generic Views
- Django - Form Processing
- Django - File Uploading
- Django - Apache Setup
- Django - Cookies Handling
- Django - Sessions
- Django - Caching
- Django - Comments
- Django - RSS
- Django - AJAX
- Django Useful Resources
- Django - Quick Guide
- Django - Useful Resources
- Django - Discussion
Django – MVT Architecture
Most of the web frameworks implement the MVC (Model-View-Controller) architecture. Django uses a variation of MVC and calls it the MVT (stands for Model-View-Template) architecture.
The Advantage of Using a Web Framework
A software framework, in general, is a standard, reusable software platform that facilitates rapid development of software applications. In contrast, a web framework (also called web application framework) such as Django provides a generic functionality needed for building web applications, APIs and web services.
The main advantage of employing a web framework is that it provides out-of-the-box support to perform common operations in the process of web development. For example, you can easily connect your application to the databases.
Usually, the framework handles tasks such as session management much more efficiently. Likewise, it integrates with templating tools to render dynamic content on web page.
The MVC Architecture
This design pattern separates the entire process of web application development in three layers. The following diagram explains the interplay of these three layers.
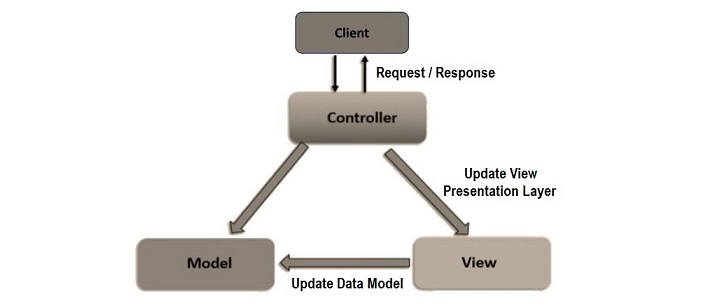
In the MVC approach, the user requests are intercepted by the controller. It coordinates with the view layer and the model layer to send the appropriate response back to the client.
The Model Layer
The Model is known as the lowest level which means it is responsible for maintaining the data. It handles data.
The model layer is connected to the database. It responds to the controller requests because the controller never talks to the database by itself. The model talks to the database back and forth and then it gives the needed data to the controller.
The model is responsible for data definitions, its processing logic and interaction with the backend database.
The View Layer
The View is the presentation layer of the application. It takes care of the placement and formatting of the result and sends it to the controller, which in turn, redirects it to the client as the application’s response.
Data representation is done by the view component. It actually generates the UI or user interface for the user. So, at web applications, when you think of the View component, just think the HTML/CSS part.
Views are created by the data which is collected by the model component but these data aren’t taken directly but through the controller, so the view only speaks to the controller.
The Controller Layer
It coordinates with the View layer and the model layer to send the appropriate response back to the client.
The Controller layer receives the request from the client, and forwards it to the model layer. The Model layer updates the data and sends back to the controller. The Controller updates the view and sends back the response to the user.
The MVT Architecture
The Django framework adapts a MVT approach. It is a slight variation of the MVC approach. The acronym MVT stands for Model, View and Template.
Here too, the Model is the data layer of the application. The View is in fact the layer that undertakes the processing logic. The Template is the presentation layer.
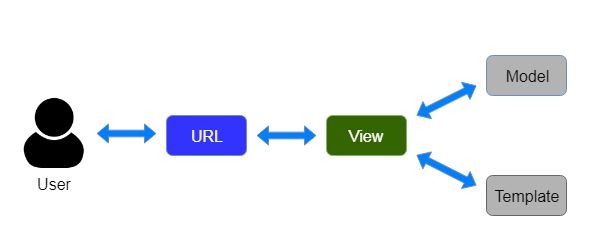
Components of a Django Application
A Django application consists of the following components −
- URL dispatcher
- View
- Model
- Template
The URL Dispatcher
Django’s URL dispatcher mechanism is equivalent to Controller in the MVC architecture. The urls.py module in the Django project’s package folder acts as the dispatcher. It defines the URL patterns. Each URL pattern is mapped with a view function to be invoked when the client’s request URL is found to be matching with it.
The URL patterns defined in each app under the project are also included in it.
When the server receives a request in the form of client URL, the dispatcher matches its pattern with the patterns available in the urls.py and routes the flow of the application towards its associated view.
The View Function
The View function reads the path parameters, query parameters and the body parameters included in the client’s request. It uses this data to interact with the models to perform CRUD operations, if required.
The Model Class
A Model is a Python class. Django uses the attributes of the Model class to construct a database table of a matching structure. Django’s Object Relational Mapper helps in performing CRUD operations in an object oriented way instead of invoking SQL queries.
The View uses the data from the client as well as the model and renders its response in the form of a template.
Template
A Template is a web page in which HTML script is interspersed with the code blocks of Django Template Language.
Django’s template processor uses any context data received from the View is inserted in these blocks so that a dynamic response is formulated. The View in turn returns the response to the user.
This is how Django’s MVT architecture handles the request-response cycle in a web application.
To Continue Learning Please Login