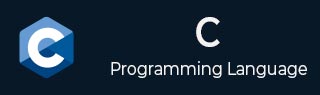
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Memory Address
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Increment and Decrement Operators in C
C - Increment and Decrement Operators
The increment operator (++) increments the value of a variable by 1, while the decrement operator (--) decrements the value.
Increment and decrement operators are frequently used in the construction of counted loops in C (with the for loop). They also have their application in the traversal of array and pointer arithmetic.
The ++ and -- operators are unary and can be used as a prefix or posfix to a variable.
Example of Increment and Decrement Operators
The following example contains multiple statements demonstrating the use of increment and decrement operators with different variations −
#include <stdio.h> int main() { int a = 5, b = 5, c = 5, d = 5; a++; // postfix increment ++b; // prefix increment c--; // postfix decrement --d; // prefix decrement printf("a = %d\n", a); printf("b = %d\n", b); printf("c = %d\n", c); printf("d = %d\n", d); return 0; }
Output
When you run this code, it will produce the following output −
a = 6 b = 6 c = 4 d = 4
Example Explanation
In other words, "a++" has the same effect as "++a", as both the expressions increment the value of variable "a" by 1. Similarly, "a--" has the same effect as "--a".
The expression "a++;" can be treated as the equivalent of the statement "a = a + 1;". Here, the expression on the right adds 1 to "a" and the result is assigned back to 1, therby the value of "a" is incremented by 1.
Similarly, "b--;" is equivalent to "b = b – 1;".
Types of Increment Operator
There are two types of increment operators – pre increment and post increment.
Pre (Prefix) Increment Operator
In an expression, the pre-increment operator increases the value of a variable by 1 before the use of the value of the variable.
Syntax
++variable_name;
Example
In the following example, we are using a pre-increment operator, where the value of "x" will be increased by 1, and then the increased value will be used in the expression.
#include <stdio.h> int main() { int x = 10; int y = 10 + ++x; printf("x = %d, y = %d\n", x, y); return 0; }
When you run this code, it will produce the following output −
x = 11, y = 21
Post (Postfix) Increment Operator
In an expression, the post-increment operator increases the value of a variable by 1 after the use of the value of the variable.
Syntax
variable_name++;
Example
In the following example, we are using post-increment operator, where the value of "x" will be used in the expression and then it will be increased by 1.
#include <stdio.h> int main() { int x = 10; int y = 10 + x++; printf("x = %d, y = %d\n", x, y); return 0; }
When you run this code, it will produce the following output −
x = 11, y = 20
Types of Decrement Operator
There are two types of decrement operators – pre decrement and post decrement.
Pre (Prefix) decrement Operator
In an expression, the pre-decrement operator decreases the value of a variable by 1 before the use of the value of the variable.
Syntax
--variable_name;
Example
In the following example, we are using a pre-decrement operator, where the value of "x" will be decreased by 1, and then the decreased value will be used in the expression.
#include <stdio.h> int main() { int x = 10; int y = 10 + --x; printf("x = %d, y = %d\n", x, y); return 0; }
When you run this code, it will produce the following output −
x = 9, y = 19
Post (Postfix) Decrement Operator
In an expression, the post-decrement operator decreases the value of a variable by 1 after the use of the value of the variable.
Syntax
variable_name--;
Example
In the following example, we are using post-decrement operator, where the value of "x" will be used in the expression and then it will be decreased by 1.
#include <stdio.h> int main() { int x = 10; int y = 10 + x--; printf("x = %d, y = %d\n", x, y); return 0; }
When you run this code, it will produce the following output −
x = 9, y = 20
More Examples of Increment and Decrement Operators
Example 1
The following example highlights the use of prefix/postfix increment/decrement −
#include <stdio.h> int main(){ char a = 'a', b = 'M'; int x = 5, y = 23; printf("a: %c b: %c\n", a, b); a++; printf("postfix increment a: %c\n", a); ++b; printf("prefix increment b: %c\n", b); printf("x: %d y: %d\n", x, y); x--; printf("postfix decrement x : %d\n", x); --y; printf("prefix decrement y : %d\n", y); return 0; }
Output
When you run this code, it will produce the following output −
a: a b: M postfix increment a: b prefix increment b: N x: 5 y: 23 postfix decrement x: 4 prefix decrement y: 22
The above example shows that the prefix as well as postfix operators have the same effect on the value of the operand variable. However, when these "++" or "--" operators appear along with the other operators in an expression, they behave differently.
Example 2
In the following code, the initial values of "a" and "b" variables are same, but the printf() function displays different values −
#include <stdio.h> int main(){ int x = 5, y = 5; printf("x: %d y: %d\n", x,y); printf("postfix increment x: %d\n", x++); printf("prefix increment y: %d\n", ++y); return 0; }
Output
Run the code and check its output −
x: 5 y: 5 postfix increment x: 5 prefix increment y: 6
In the first case, the printf() function prints the value of "x" and then increments its value. In the second case, the increment operator is executed first, the printf() function uses the incremented value for printing.
Operator Precedence of Increment and Decrement Operators
There are a number of operators in C. When multiple operators are used in an expression, they are executed as per their order of precedence. Increment and decrement operators behave differently when used along with other operators.
When an expression consists of increment or decrement operators alongside other operators, the increment and decrement operations are performed first. Postfix increment and decrement operators have higher precedence than prefix increment and decrement operators.
Read also: Operator Precedence in C
Example 1
Take a look at the following example −
#include <stdio.h> int main(){ int x = 5, z; printf("x: %d \n", x); z = x++; printf("x: %d z: %d\n", x, z); return 0; }
Output
Run the code and check its output −
x: 5 x: 6 z: 5
Since "x++" increments the value of "x" to 6, you would expect "z" to be 6 as well. However, the result shows "z" as 5. This is because the assignment operator has a higher precedence over postfix increment operator. Hence, the existing value of "x" is assigned to "z", before incrementing "x".
Example 2
Take a look at another example below −
#include <stdio.h> int main(){ int x = 5, y = 5, z; printf("x: %d y: %d\n", x,y); z = ++y; printf("y: %d z: %d\n", y ,z); return 0; }
Output
When you run this code, it will produce the following output −
y: 5 y: 6 z: 6
The result may be confusing, as the value of "y" as well as "z" is now 6. The reason is that the prefix increment operator has a higher precedence than the assignment operator. Hence, "y" is incremented first and then its new value is assigned to "z".
The associativity of operators also plays an important part. For increment and decrement operators, the associativity is from left to right. Hence, if there are multiple increment or decrement operators in a single expression, the leftmost operator will be executed first, moving rightward.
Example 3
In this example, the assignment expression contains both the prefix as well as postfix operators.
#include <stdio.h> int main(){ int x = 5, y = 5, z; z = x++ + ++y; printf("x: %d y: %d z: %d\n", x,y,z); return 0; }
Output
Run the code and check its output −
x: 6 y:6 z: 11
In this example, the first operation to be done is "y++" ("y" becomes 6). Secondly the "+" operator adds "x" (which is 5) and "y", the result assigned to "z" as 11, and then "x++" increments "x" to 6.
Using the Increment Operator in Loop
In C, the most commonly used syntax of a for loop is as follows −
for (init_val; final_val; increment) { statement(s); }
Example
The looping body is executed for all the values of a variable between the initial and the final values, incrementing it after each round.
#include <stdio.h> int main(){ int x; for (x = 1; x <= 5; x++){ printf("x: %d\n", x); } return 0; }
Output
When you run this code, it will produce the following output −
x: 1 x: 2 x: 3 x: 4 x: 5
To Continue Learning Please Login