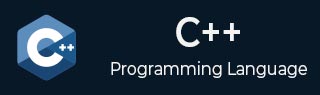
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C++ Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
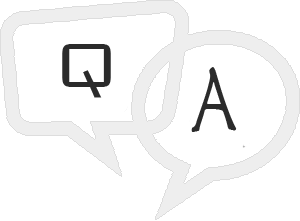
Answer : C
Explaination
Few characters have alternative representation and start with ??. Eg. Fro [ equivalent is ??(
Q 2 - What is the output of the following program?
#include<iostream> using namespace std; class abc { public: int i; abc(int i) { i = i; } }; main() { abc m(5); cout<<m.i; }
Answer : B
Explaination
i=i, is assigning member variable to itself.
#include<iostream> using namespace std; class abc { public: int i; abc(int i) { i = i; } }; main() { abc m(5); cout<<m.i; }
Q 3 - In the following program f() is overloaded.
void f(int x) { } void f(signed x) { } main() { }
Answer : B
Explaination
No, as both the functions signature is same.
Q 4 - What is the output of the following program?
#include<iostream> using namespace std; class Base { public: virtual void f() { cout<<"Base\n"; } }; class Derived:public Base { public: void f() { cout<<"Derived\n"; } }; main() { Base *p = new Derived(); p->f(); }
Answer : B
Explaination
The overridden method f() of the created object for derived class gets called.
#include<iostream> using namespace std; class Base { public: virtual void f() { cout<<"Base\n"; } }; class Derived:public Base { public: void f() { cout<<"Derived\n"; } }; main() { Base *p = new Derived(); p->f(); }
Q 5 - Which feature of the OOPS gives the concept of reusability?
Answer : C
Explaination
The process of designing a new class (derived) from the existing (base) class to acquire the attributes of the existing is called as inheritance. Inheritance gives the concept of reusability for code/software components.
Q 6 - i) single file can be opened by several streams simultaneously.
ii) several files simultaneously can be opened by a single stream
Answer : C
Explaination
Q 7 - i) Exception handling technically provides multi branching.
ii) Exception handling can be mimicked using ‘goto’ construct.
Answer : A
Explaination
goto just does the unconditional branching.
Q 8 - What is the output of the following program?
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Answer : B
Explaination
5 3, call by value mechanism can’t alter actual arguments.
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Q 9 - What is the output of the following program?
#include<iostream> using namespace std; int x = 5; int &f() { return x; } main() { f() = 10; cout<<x; }
Answer : D
Explaination
A function can return reference, hence it can appear on the left hand side of the assignment operator.
#include<iostream> using namespace std; int x = 5; int &f() { return x; } main() { f() = 10; cout<<x; }
Q 10 - What is the output of the following program?
#include<iostream> using namespace std; main() { int a[] = {10, 20, 30}; cout<<*a+1; }
Answer : C
Explaination
*a refers to 10 and adding a 1 to it gives 11.
#include<iostream> using namespace std; main() { int a[] = {10, 20, 30}; cout<<*a+1; }
To Continue Learning Please Login