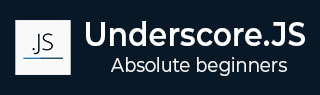
- Underscore.JS Tutorial
- Underscore.JS - Home
- Underscore.JS - Overview
- Underscore.JS - Environment Setup
- Underscore.JS - Iterating Collection
- Underscore.JS - Processing Collection
- Underscore.JS - Iterating Array
- Underscore.JS - Processing Array
- Underscore.JS - Functions
- Underscore.JS - Mapping Objects
- Underscore.JS - Updating Objects
- Underscore.JS - Comparing Objects
- Underscore.JS - Utilities
- Underscore.JS - Chaining
- Underscore.JS Useful Resources
- Underscore.JS - Quick Guide
- Underscore.JS - Useful Resources
- Underscore.JS - Discussion
Underscore.JS - memoize method
Syntax
_.memoize(function, [hashFunction])
memoize method speeds up the slow computation. It remembers the a given function by caching its output. hashFunction if passed is used to compute the hash value to store the result based on arguments passed to original function. See the below example
Example
var _ = require('underscore'); var fibonacci = _.memoize(function(n) { return n < 2 ? n: fibonacci(n - 1) + fibonacci(n - 2); }); var fibonacci1 = function(n) { return n < 2 ? n: fibonacci1(n - 1) + fibonacci1(n - 2); }; var startTimestamp = new Date().getTime(); var result = fibonacci(1000); var endTimestamp = new Date().getTime(); console.log(result + " in " + ((endTimestamp - startTimestamp)) + ' ms'); startTimestamp = new Date().getTime(); result = fibonacci1(30); endTimestamp = new Date().getTime(); console.log(result + " in " + ((endTimestamp - startTimestamp)) + ' ms');
Save the above program in tester.js. Run the following command to execute this program.
Command
\>node tester.js
Output
4.346655768693743e+208 in 6 ms 832040 in 30 ms
underscorejs_functions.htm
Advertisements
To Continue Learning Please Login