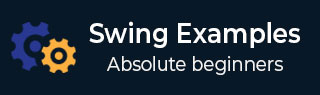
- Swing Programming Examples
- Example - Home
- Example - Environment Setup
- Example - Borders
- Example - Buttons
- Example - CheckBoxes
- Example - Combo Boxes
- Example - Color Choosers
- Example - Dialogs
- Example - Editor Panes
- Example - File Choosers
- Example - Formatted TextFields
- Example - Frames
- Example - Lists
- Example - Layouts
- Example - Menus
- Example - Password Fields
- Example - Progress Bars
- Example - Scroll Panes
- Example - Sliders
- Example - Spinners
- Example - Tables
- Example - Toolbars
- Example - Tree
- Swing Useful Resources
- Swing - Quick Guide
- Swing - Useful Resources
- Swing - Discussion
Swing Examples - Respond to window's event
Following example showcase how to respond to window's event in Swing based application.
We can use the following option when user click the close button of the frame.
DO_NOTHING_ON_CLOSE − No action. Just listen the windowClosing event to do further action.
HIDE_ON_CLOSE − This is the default behaviour of JFrame and JDialog to hide when close button is clicked.
DISPOSE_ON_CLOSE − Hides and close the window and frees up any resources used by the window.
EXIT_ON_CLOSE − Exits the application using System.exit(0).
Following example show cases the use of DO_NOTHING_ON_CLOSE.
Example
import java.awt.BorderLayout; import java.awt.FlowLayout; import java.awt.LayoutManager; import java.awt.event.WindowEvent; import java.awt.event.WindowListener; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; public class SwingTester { public static void main(String[] args) { createWindow(); } private static void createWindow() { JFrame frame = new JFrame("Swing Tester"); frame.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE); frame.addWindowListener(new WindowListener() { public void windowOpened(WindowEvent e) {} public void windowIconified(WindowEvent e) {} public void windowDeiconified(WindowEvent e) {} public void windowDeactivated(WindowEvent e) {} public void windowClosing(WindowEvent e) { System.exit(0); } public void windowClosed(WindowEvent e) {} public void windowActivated(WindowEvent e) {} }); createUI(frame); frame.setSize(560, 200); frame.setLocationRelativeTo(null); frame.setVisible(true); } private static void createUI(JFrame frame){ JPanel panel = new JPanel(); LayoutManager layout = new FlowLayout(); panel.setLayout(layout); panel.add(new JLabel("Hello World")); frame.getContentPane().add(panel, BorderLayout.CENTER); } }
Output
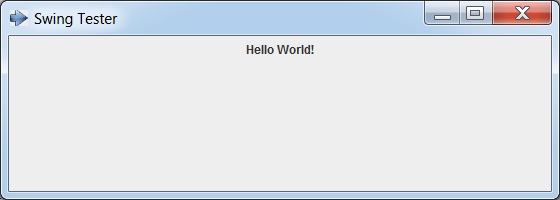
swingexamples_frames.htm
Advertisements
To Continue Learning Please Login