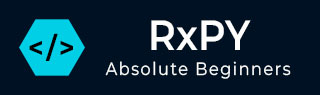
- RxPY Tutorial
- RxPY - Home
- RxPY - Overview
- RxPY - Environment Setup
- RxPY - Latest Release Updates
- RxPY - Working with Observables
- RxPY- Operators
- RxPY - Working With Subject
- RxPY - Concurrency Using Scheduler
- RxPY - Examples
- RxPY Useful Resources
- RxPY - Quick Guide
- RxPY - Useful Resources
- RxPY - Discussion
RxPY - Conditional and Boolean Operators
all
This operator will check if all the values from the source observable satisfy the condition given.
Syntax
all(predicate)
Parameters
predicate: boolean. This function will be applied to all the values, from the source observable and will return true or false based on the condition given.
Return value
The return value is an observable, which will have the boolean value true or false, based on the condition applied on all the values of the source observable.
Example 1
from rx import of, operators as op test = of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) sub1 = test.pipe( op.all(lambda a: a<10) ) sub1.subscribe(lambda x: print("The result is {0}".format(x)))
Output
E:\pyrx>python testrx.py The result is False
Example 2
from rx import of, operators as op test = of(1, 2, 3, 4, 5, 6, 7, 8, 9) sub1 = test.pipe( op.all(lambda a: a<10) ) sub1.subscribe(lambda x: print("The result is {0}".format(x)))
Output
E:\pyrx>python testrx.py The result is True
contains
This operator will return an observable with the value true or false if the given value is present is the values of the source observable.
Syntax
contains(value, comparer=None)
Parameters
value: The value to be checked if present in the source observable
comparer: optional. This is a comparer function to be applied to the values present in the source observable for comparison.
Example
from rx import of, operators as op test = of(17, 25, 34, 56, 78) sub1 = test.pipe( op.contains(34) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is True
Example 2: Using comparer
from rx import of, operators as op test = of(17, 25, 34, 56, 78) sub1 = test.pipe( op.contains(34, lambda x, y: x == y) ) sub1.subscribe(lambda x: print("The valus is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is True
default_if_empty
This operator will return a default value if the source observable is empty.
Syntax
default_if_empty(default_value=None)
Parameters
default_value: optional. It will give the output, as None is nothing is passed as default_value, else it will give whatever value passed.
Return value
It will return an observable with a default value if the source observable is empty.
Example 1
from rx import of, operators as op test = of() sub1 = test.pipe( op.default_if_empty() ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is None
Example 2: default_value passed
from rx import of, operators as op test = of() sub1 = test.pipe( op.default_if_empty("Empty!") ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is Empty!
sequence_equal
This operator will compare two sequences of observables, or an array of values and return an observable with the value true or false.
Syntax
sequence_equal(second_seq, comparer=None)
Parameters
second_seq: observable or array to be compared with the first observable.
comparer: optional. Comparer function to be applied to compare values in both sequences.
Example
from rx import of, operators as op test = of(1,2,3) test1 = of(1,2,3) sub1 = test.pipe( op.sequence_equal(test1) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is True
Example: using a comparer function
from rx import of, operators as op test = of(1,2,3) test1 = of(1,2,3) sub1 = test.pipe( op.sequence_equal(test1, lambda x, y : x == y) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is True
skip_until
This operator will discard values from the source observable until the second observable emits a value.
Syntax
skip_until(observable)
Parameters
observable: the second observable which when emits a value will trigger the source observable.
Return value
It will return an observable which will have values from the source observable until the second observable emits a value.
Example
from rx import interval,range, operators as op from datetime import date test = interval(0) test1 = range(10) sub1 = test1.pipe( op.skip_until(test) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 0 The value is 1 The value is 2 The value is 3 The value is 4 The value is 5 The value is 6 The value is 7 The value is 8 The value is 9
skip_while
This operator will return an observable with values from the source observable that satisfies the condition passed.
Syntax
skip_while(predicate_func)
Parameters
predicate_func: This function will be applied to all the values of the source observable, and return the values which satisfy the condition.
Return value
It will return an observable with values from the source observable that satisfies the condition passed.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.skip_while(lambda x : x < 5) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 5 The value is 6 The value is 7 The value is 8 The value is 9 The value is 10
take_until
This operator will discard values from the source observable after the second observable emits a value or is terminated.
Syntax
take_until(observable)
Parameters
observable: the second observable which, when emits a value will terminate the source observable.
Return value
It will return an observable, which will have values from the source observable only, when the second observable used emits a value.
Example
from rx import timer,range, operators as op from datetime import date test = timer(0.01) test1 = range(500) sub1 = test1.pipe( op.take_until(test) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
In this example, you will get the values emitted from range. But, once the timer is done, it will stop the source observable from emitting further.
Output
E:\pyrx>python testrx.py The value is 0 The value is 1 The value is 2 The value is 3 The value is 4 The value is 5 The value is 6 The value is 7 The value is 8 The value is 9 The value is 10 The value is 11 The value is 12 The value is 13 The value is 14 The value is 15 The value is 16 The value is 17 The value is 18 The value is 19 The value is 20 The value is 21 The value is 22 The value is 23 The value is 24 The value is 25 The value is 26
take_while
This operator will discard values from the source observable when the condition fails.
Syntax
take_while(predicate_func)
Parameters
predicate_func: this function will evaluate each value of the source observable.
Return value
It will return an observable with values till the predicate function satisfies.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.take_while(lambda a : a < 5) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 1 The value is 2 The value is 3 The value is 4