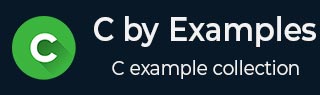
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Swapping Two Numbers Without Variable
In many case, programmers are required to swap values of two variables. Here, we shall learn how to swap values of two integer variables, that may lead to swapping of values of any type. Values between variables can be swapped in two ways −
- With help of a third (temp) variable
- Without using any temporary variable
We have already learnt the first method. Here we shall learn the second method. Though it looks like a magic but it is just a small trick. Imagine we have two number 1 and 2 stored in a and b respectively now −
If we add a and b (1 + 2) and store it to a then a will become 3 and b is still 2.
Now we subtract b (2) from new value of a (3) and store it to b then a is still 3 and b becomes 1. Note that 1 was earlier hold by a.
Now we subtract b which has new value (1) from a (3) and store it to b ( a = 3 - 1) then a holds the value 2 which was earlier hold by b.
Conclusion − values are swapped.
Algorithm
We shall now define the above mentioned procedure in step-by-step algorithmic manner −
START Var1, Var2 Step 1 → Add Var1 and Var2 and store to Var1 Step 2 → Subtract Var2 from Var1 and store to Var2 Step 3 → Subtract Var2 from Var1 and store to Var1 STOP
Pseudocode
From the above algorithm, we can draw pseudocode for this program −
procedure swap(a, b) a ← a + b // a holds the sum of both b ← a - b // b now holds the value of a a ← a - b // a now holds value of b end procedure
Implementation
C implementation of the above algorithm should look like this −
#include <stdio.h> int main() { int a, b; a = 11; b = 99; printf("Values before swapping - \n a = %d, b = %d \n\n", a, b); a = a + b; // ( 11 + 99 = 110) b = a - b; // ( 110 - 99 = 11) a = a - b; // ( 110 - 11 = 99) printf("Values after swapping - \n a = %d, b = %d \n", a, b); }
Output
Output of this program should be −
Values before swapping - a = 11, b = 99 Values after swapping - a = 99, b = 11