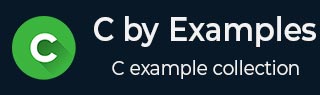
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Program To Find HCF In C
An H.C.F or Highest Common Factor, is the largest common factor of two or more values.
For example factors of 12 and 16 are −
12 → 1, 2, 3, 4, 6, 12
16 → 1, 2, 4, 8, 16
The common factors are 1, 2, 4 and the highest common factor is 4.
Algorithm
Algorithm of this program can be derived as −
START Step 1 → Define two variables - A, B Step 2 → Set loop from 1 to max of A, B Step 3 → Check if both are completely divided by same loop number, if yes, store it Step 4 → Display the stored number is HCF STOP
Pseudocode
procedure even_odd() Define two variables a and b FOR i = 1 TO MAX(a, b) DO IF a % i is 0 AND b % i is 0 THEN HCF = i ENDIF ENDFOR DISPLAY HCF end procedure
Implementation
Implementation of this algorithm is given below −
#include<stdio.h> int main() { int a, b, i, hcf; a = 12; b = 16; for(i = 1; i <= a || i <= b; i++) { if( a%i == 0 && b%i == 0 ) hcf = i; } printf("HCF = %d", hcf); return 0; }
Output
Output of the program should be −
HCF = 4
mathematical_programs_in_c.htm
Advertisements