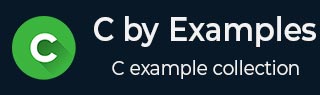
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Program to divide array
To divide an array into two, we need at least three array variables. We shall take an array with continuous numbers and then shall store the values of it into two different variables based on even and odd values.
Algorithm
Let's first see what should be the step-by-step procedure of this program −
START Step 1 → Take three array variables A, E, and O Step 2 → Store continuous values in A Step 3 → Loop for each value of A Step 4 → If A[n] is even then store in E array Step 5 → If A[n] is odd then store in E array STOP
Pseudocode
Let's now see the pseudocode of this algorithm −
procedure divide_array(A) FOR EACH value in A DO IF A[n] is even save in E ELSE save in O END IF END FOR end procedure
Implementation
The implementation of the above derived pseudocode is as follows −
#include <stdio.h> int main() { int array[10] = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9}; int even[10], odd[10]; int loop, e, d; e = d = 0; for(loop = 0; loop < 10; loop++) { if(array[loop]%2 == 0) { even[e] = array[loop]; e++; } else { odd[d] = array[loop]; d++; } } printf(" original -> "); for(loop = 0; loop < 10; loop++) printf(" %d", array[loop]); printf("\n even -> "); for(loop = 0; loop < e; loop++) printf(" %d", even[loop]); printf("\n odd -> "); for(loop = 0; loop < d; loop++) printf(" %d", odd[loop]); return 0; }
The output should look like this −
original -> 0 1 2 3 4 5 6 7 8 9 even -> 0 2 4 6 8 odd -> 1 3 5 7 9
array_examples_in_c.htm
Advertisements