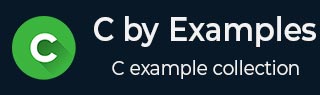
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Program to copy array in C
This program shall help you learn one of basics of arrays. Copying an array involves index-by-index copying. For this to work we shall know the length of array in advance, which we shall use in iteration. Another array of same length shall be required, to which the array will be copied.
Algorithm
Let's first see what should be the step-by-step procedure of this program −
START Step 1 → Take two arrays A, B Step 2 → Store values in A Step 3 → Loop for each value of A Step 4 → Copy each index value to B array at the same index location STOP
Pseudocode
Let's now see the pseudocode of this algorithm −
procedure copy_array(A, B) SET index to 1 FOR EACH value in A DO B[index] = A[index] INCREMENT index END FOR end procedure
Implementation
The implementation of the above derived pseudocode is as follows −
#include <stdio.h> int main() { int original[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}; int copied[10]; int loop; for(loop = 0; loop < 10; loop++) { copied[loop] = original[loop]; } printf("original -> copied \n"); for(loop = 0; loop < 10; loop++) { printf(" %2d %2d\n", original[loop], copied[loop]); } return 0; }
The output should look like this −
original -> copied 1 1 2 2 3 3 4 4 5 5 6 6 7 7 8 8 9 9 0 0
array_examples_in_c.htm
Advertisements