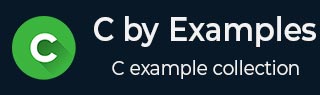
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Armstrong Number Program In C
An armstrong number is a number which equal to the sum of the cubes of its individual digits. For example, 153 is an armstrong number as −
153 = (1)3 + (5)3 + (3)3 153 = 1 + 125 + 27 153 = 153
Algorithm
Algorithm of this program is very easy −
START Step 1 → Take integer variable Arms Step 2 → Assign value to the variable Step 3 → Split all digits of Arms Step 4 → Find cube-value of each digits Step 5 → Add all cube-values together Step 6 → Save the output to Sum variable Step 7 → If Sum equals to Arms print Armstrong Number Step 8 → If Sum not equals to Arms print Not Armstrong Number STOP
Pseudocode
We can draft a pseudocode of the above algorithm as follows −
procedure armstrong : number check = number rem = 0 WHILE check IS NOT 0 rem ← check modulo 10 sum ← sum + (rem)3 divide check by 10 END WHILE IF sum equals to number PRINT armstrong ELSE PRINT not an armstrong END IF end procedure
Implementation
Implementation of this algorithm is given below. You can change the value of arms
variable and execute and check your program −
#include <stdio.h> int main() { int arms = 153; int check, rem, sum = 0; check = arms; while(check != 0) { rem = check % 10; sum = sum + (rem * rem * rem); check = check / 10; } if(sum == arms) printf("%d is an armstrong number.", arms); else printf("%d is not an armstrong number.", arms); return 0; }
Output
Output of the program should be −
153 is an armstrong number.
mathematical_programs_in_c.htm
Advertisements