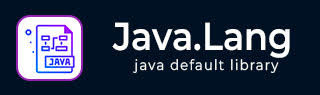
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StringBuilder codePointCount() Method
The Java StringBuilder codePointCount() method returns the number of Unicode code points in the specified text range of a StingBuilder object.
The text range begins at the specified beginIndex and extends to the char at index endIndex - 1. Therefore the length (in chars) of the text range is endIndex – beginIndex. A code point is a numerical value that maps to a specific character.
The codePointCount() method accepts two parameters as an integer value that holds the value of the beginIndex and endIndex. It throws an exception if the beginIndex value is negative or the endIndex value is greater than the sequence length.
Syntax
Following is the syntax of the Java StringBuilder codePointCount() method −
public int codePointCount(int beginIndex, int endIndex)
Parameters
beginIndex − This is the index to the first char of the text range.
endIndex − This is the index after the last char of the text range.
Return Value
This method returns the number of Unicode code points in the specified text range.
Example
If the beginIndex value is greater than 0 and the endIndex value is less than the sequence length, it returns the character code point count within the given range.
In the following program, we are creating a StringBuilder with the value “programming”. Using the codePointCount() method, we are trying to count the character code point within the given range.
package com.tutorialspoint.StringBuilder; import java.lang.*; public class StringBuilderDemo { public static void main(String[] args) { //create an object of the StringBuilder StringBuilder sb = new StringBuilder("programming"); System.out.println("String is: " + sb); //initialize the beginIndex and endIndex int beginIndex = 1; int endIndex = 5; System.out.println("The begin and end index values are: " + beginIndex + " and " + endIndex); //using the codePointCount() method int count = sb.codePointCount(beginIndex, endIndex); System.out.println("The character code point count between " + beginIndex + " and " + endIndex + " is: " + count); } }
Output
On executing the above program, it will produce the following result −
String is: programming The begin and end index values are: 1 and 5 The character code point count between 1 and 5 is: 4
Example
If the beginIndex value is negative, it throws an IndexOutOfBoundsException, when we invoke the method on it.
In the following example, we are creating an object of the StringBuilder with the value “TutorialsPoint”. Using the codePointCount() method, we are trying to count the character code point at the beginning index -1 and till the ending index 8.
package com.tutorialspoint.StringBuilder; public class Demo { public static void main(String[] args) { try { //create an object of the StringBuilder StringBuilder sb = new StringBuilder("TutorialsPoint"); System.out.println("String is: " + sb); //initialize the beginIndex and endIndex int beginIndex = -1; int endIndex = 8; System.out.println("The beginIndex and endIndex values are: " + beginIndex + " and " + endIndex); //using the codePointCount() method int count = sb.codePointCount(beginIndex, endIndex); System.out.println("The character code point count between " + beginIndex + " and " + endIndex + " is: " + count); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
Output
Following is the output of the above program −
String is: TutorialsPoint The beginIndex and endIndex values are: -1 and 8 java.lang.IndexOutOfBoundsException at java.base/java.lang.AbstractStringBuilder.codePointCount(AbstractStringBuilder.java:443) at java.base/java.lang.StringBuilder.codePointCount(StringBuilder.java:91) at com.tutorialspoint.StringBuilder.Demo.main(Demo.java:13) Exception: java.lang.IndexOutOfBoundsException
Example
If the endIndex value is greater than the sequence length, the codePointCount() method throws an IndexOutOfBoundsException.
In this program, we are instantiating the StringBuilder class with the value “WelcomeIndia”. Then, using the codePointCount() method, we are trying to count the character code point at the beginning index 1 to till the ending index whose value is greater than the sequence length.
package com.tutorialspoint.StringBuilder; public class Test { public static void main(String[] args) { try { //create an object of the StringBuilder StringBuilder sb = new StringBuilder("WelcomeIndia"); System.out.println("String is: " + sb); //initialize the beginIndex and endIndex int beginIndex = 1; int endIndex = 30; System.out.println("The beginIndex and endIndex values are: " + beginIndex + " and " + endIndex); //using the codePointCount() method int count = sb.codePointCount(beginIndex, endIndex); System.out.println("The character code point count between " + beginIndex + " and " + endIndex + " is: " + count); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
Output
The above program, produces the following results −
String is: WelcomeIndia The beginIndex and endIndex values are: 1 and 30 java.lang.IndexOutOfBoundsException at java.base/java.lang.AbstractStringBuilder.codePointCount(AbstractStringBuilder.java:443) at java.base/java.lang.StringBuilder.codePointCount(StringBuilder.java:91) at com.tutorialspoint.StringBuilder.Test.main(Test.java:13) Exception: java.lang.IndexOutOfBoundsException